帮我写一个部门员工管理系统
时间: 2023-08-20 17:28:44 浏览: 115
好的,部门员工管理系统可以分为前端界面和后端数据处理两部分。下面是一个简单的部门员工管理系统的示例代码,包括前端和后端的实现。
前端界面代码:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>部门员工管理系统</title>
<style>
table {
border-collapse: collapse;
}
th, td {
padding: 10px;
border: 1px solid black;
}
</style>
</head>
<body>
<h1>部门员工管理系统</h1>
<h2>部门列表</h2>
<table>
<thead>
<tr>
<th>部门编号</th>
<th>部门名称</th>
<th>部门负责人</th>
<th>部门上级</th>
<th>部门描述</th>
<th>部门成员</th>
<th>部门创建时间</th>
<th>部门状态</th>
</tr>
</thead>
<tbody id="department-list">
</tbody>
</table>
<h2>员工列表</h2>
<table>
<thead>
<tr>
<th>员工编号</th>
<th>员工姓名</th>
<th>员工性别</th>
<th>员工年龄</th>
<th>员工职位</th>
<th>所属部门</th>
<th>员工入职时间</th>
<th>员工状态</th>
</tr>
</thead>
<tbody id="employee-list">
</tbody>
</table>
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script src="app.js"></script>
</body>
</html>
```
后端数据处理代码:
```python
from flask import Flask, jsonify, request
import json
app = Flask(__name__)
# 初始化部门和员工数据
departments = [
{
"id": 1,
"name": "技术部",
"leader": "张三",
"parent": None,
"description": "负责公司技术研发",
"members": [1001, 1002],
"create_time": "2022-01-01",
"status": "正常"
},
{
"id": 2,
"name": "市场部",
"leader": "李四",
"parent": None,
"description": "负责公司市场拓展",
"members": [1003, 1004],
"create_time": "2022-01-01",
"status": "正常"
}
]
employees = [
{
"id": 1001,
"name": "张三",
"gender": "男",
"age": 30,
"position": "技术总监",
"department": 1,
"hire_date": "2020-01-01",
"status": "在职"
},
{
"id": 1002,
"name": "李四",
"gender": "女",
"age": 25,
"position": "Java工程师",
"department": 1,
"hire_date": "2021-01-01",
"status": "在职"
},
{
"id": 1003,
"name": "王五",
"gender": "男",
"age": 35,
"position": "市场总监",
"department": 2,
"hire_date": "2019-01-01",
"status": "在职"
},
{
"id": 1004,
"name": "赵六",
"gender": "女",
"age": 28,
"position": "市场专员",
"department": 2,
"hire_date": "2020-01-01",
"status": "在职"
}
]
# 获取部门列表
@app.route('/departments')
def get_departments():
return jsonify(departments)
# 获取员工列表
@app.route('/employees')
def get_employees():
return jsonify(employees)
# 获取指定部门信息
@app.route('/departments/<int:department_id>')
def get_department(department_id):
for department in departments:
if department["id"] == department_id:
return jsonify(department)
return jsonify({"error": "Department not found"})
# 获取指定员工信息
@app.route('/employees/<int:employee_id>')
def get_employee(employee_id):
for employee in employees:
if employee["id"] == employee_id:
return jsonify(employee)
return jsonify({"error": "Employee not found"})
# 新增部门
@app.route('/departments', methods=['POST'])
def add_department():
new_department = request.json
departments.append(new_department)
return jsonify(new_department)
# 新增员工
@app.route('/employees', methods=['POST'])
def add_employee():
new_employee = request.json
employees.append(new_employee)
return jsonify(new_employee)
# 修改部门信息
@app.route('/departments/<int:department_id>', methods=['PUT'])
def update_department(department_id):
for department in departments:
if department["id"] == department_id:
department.update(request.json)
return jsonify(department)
return jsonify({"error": "Department not found"})
# 修改员工信息
@app.route('/employees/<int:employee_id>', methods=['PUT'])
def update_employee(employee_id):
for employee in employees:
if employee["id"] == employee_id:
employee.update(request.json)
return jsonify(employee)
return jsonify({"error": "Employee not found"})
# 删除部门
@app.route('/departments/<int:department_id>', methods=['DELETE'])
def delete_department(department_id):
for department in departments:
if department["id"] == department_id:
departments.remove(department)
return jsonify({"message": "Department deleted"})
return jsonify({"error": "Department not found"})
# 删除员工
@app.route('/employees/<int:employee_id>', methods=['DELETE'])
def delete_employee(employee_id):
for employee in employees:
if employee["id"] == employee_id:
employees.remove(employee)
return jsonify({"message": "Employee deleted"})
return jsonify({"error": "Employee not found"})
if __name__ == '__main__':
app.run(debug=True)
```
这是一个简单的部门员工管理系统示例,你可以根据自己的需求进行更改和优化。
阅读全文
相关推荐
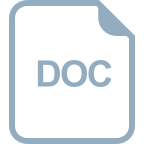
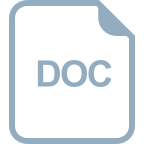
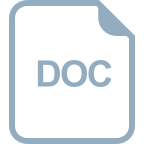
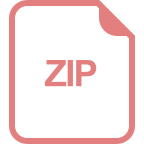
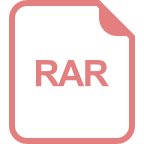
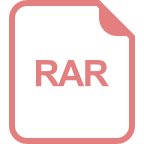
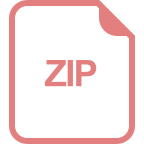
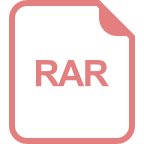
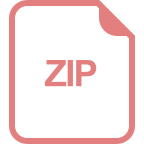
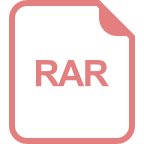
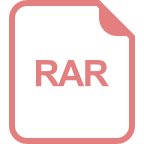
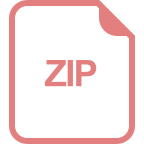
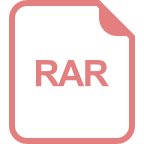
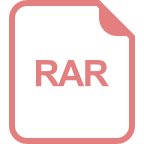
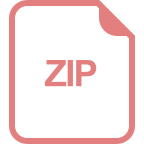
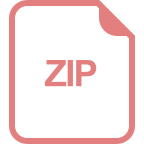
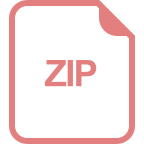
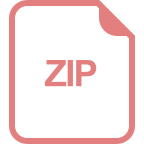
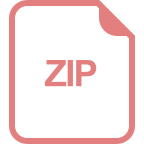