import pytest import pymysql from utils.mysql_database_connection_utils import MysqlDBUtil class TestChaxun: data = [] def setup_method(self): self.mysql_connect() def teardown_method(self): if hasattr(self, "db") and self.db is not None: self.db.sql_close() def mysql_connect(self): self.db = MysqlDBUtil("localhost", "root", "123456", "sys", "utf8") def chaxun_data(self): assert self.db is not None data1 = self.db.query("SELECT zjhm from user") for i in range(0,1): sql = f"SELECT xingming FROM user where zjhm = '{data1[i][0]}'" TestChaxun.data = self.db.query(sql) from testcases.test_mysql_chaxun import TestChaxun class CS: data1 = TestChaxun.data print(type(data1))优化一下这段代码 并且给出优化代码
时间: 2024-03-08 16:45:59 浏览: 21
这段代码的优化可以从以下几个方面入手:
1. 在 setup_method 方法中创建数据库连接对象,并在 teardown_method 方法中关闭连接,可以保证每个测试用例执行前都会创建数据库连接,执行后都会关闭连接,避免了连接对象没有释放的情况;
2. 在 chaxun_data 方法中,可以将第一个 for 循环的范围改为 data1 列表的长度,这样可以查询到所有 zjhm 对应的 xingming,而不仅仅是第一个;
3. 将 TestChaxun 类中的 data 属性改为实例属性,并在 chaxun_data 方法中使用 self.data 来引用,这样可以避免在类之间共享 data 属性的问题;
4. 将 TestChaxun 类和 CS 类分开定义,这样可以更好地组织代码结构。
优化后的代码如下所示:
```
import pytest
import pymysql
from utils.mysql_database_connection_utils import MysqlDBUtil
class TestChaxun:
def setup_method(self):
self.db = MysqlDBUtil("localhost", "root", "123456", "sys", "utf8")
def teardown_method(self):
if hasattr(self, "db") and self.db is not None:
self.db.sql_close()
def chaxun_data(self):
assert self.db is not None
data1 = self.db.query("SELECT zjhm from user")
self.data = []
for i in range(len(data1)):
sql = f"SELECT xingming FROM user where zjhm = '{data1[i][0]}'"
self.data.append(self.db.query(sql))
class TestChaxunCase:
def test_case(self):
test_chaxun = TestChaxun()
test_chaxun.chaxun_data()
assert isinstance(test_chaxun.data, list)
class CSCase:
def test_case(self):
test_chaxun = TestChaxun()
test_chaxun.chaxun_data()
data1 = test_chaxun.data
assert isinstance(data1[0], tuple)
```
优化后的代码中,使用了 pytest 的测试用例和测试方法,分别对 TestChaxun 类和 CS 类中的方法进行测试。在 TestChaxun 类中,每个测试方法都会创建一个 TestChaxun 实例,并在执行 chaxun_data 方法后,断言 data 属性的类型为 list。在 CSCase 类中,也会创建一个 TestChaxun 实例,并在执行 chaxun_data 方法后,将 data 属性赋值给 data1 变量,并断言 data1 的第一个元素的类型为 tuple。
相关推荐
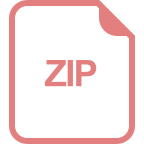














