C++代码实现替代异步co_await
时间: 2024-09-18 20:09:27 浏览: 47
在C++中,如果你想要模拟类似C#中的`co_await`语法(用于异步编程),你可以使用`std::future`或者第三方库如`boost::asio`或者更现代的`std::coroutine`。这里我将给出一个基础的例子,展示如何使用`std::async`和`std::future`来替代:
```cpp
#include <iostream>
#include <future>
// 使用future模拟异步等待
template <typename Func>
std::future<decltype(Func())> async_wait(Func&& func) {
return std::async(std::launch::async, std::forward<Func>(func));
}
void long_running_task() {
// 这里代表一个耗时的操作
std::this_thread::sleep_for(std::chrono::seconds(5));
std::cout << "Long running task finished" << std::endl;
}
int main() {
auto future_task = async_wait(long_running_task);
// 等待任务完成
while (!future_task.valid()) {
std::cout << "Waiting for task..." << std::endl;
}
try {
// 获取结果并处理
auto result = future_task.get();
// 在这里可以访问long_running_task的结果
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
```
在这个例子中,`async_wait`函数创建了一个异步操作,并返回一个`std::future`。主程序循环检查未来是否准备好,然后获取并处理结果。
阅读全文
相关推荐
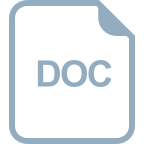
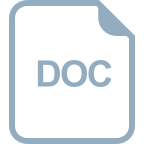
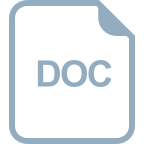
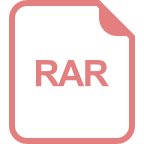
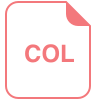
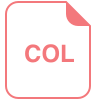
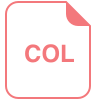
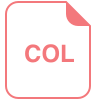
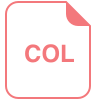
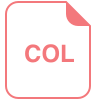
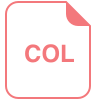
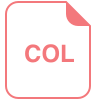
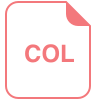
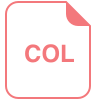
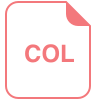
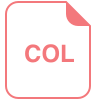
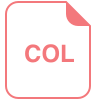
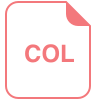
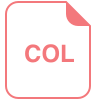