基于 AES 算法的 CBC 工作模式可视化演示程序实现,使用python语言,用tkinter实现,请写好注释。
时间: 2023-06-11 16:09:48 浏览: 47
以下是基于AES算法的CBC工作模式可视化演示程序实现的Python代码。注释已经尽可能详细,如有不懂的地方,可以提问。
``` python
from Crypto.Cipher import AES # 导入AES模块
from Crypto.Util.Padding import pad # 导入填充模块
import tkinter as tk # 导入tkinter模块
class CBC_GUI:
def __init__(self, master):
# 初始化GUI界面
self.master = master
self.master.title("基于AES算法的CBC工作模式可视化演示程序")
self.master.geometry("500x400")
# 初始化变量
self.key = tk.StringVar()
self.iv = tk.StringVar()
self.plaintext = tk.StringVar()
self.ciphertext = tk.StringVar()
# 创建界面
self.create_widgets()
def create_widgets(self):
# 创建标签和输入框
tk.Label(self.master, text="密钥(16/24/32字节):").grid(row=0, column=0, pady=10)
tk.Entry(self.master, textvariable=self.key, width=50).grid(row=0, column=1, pady=10)
tk.Label(self.master, text="初始向量(16字节):").grid(row=1, column=0, pady=10)
tk.Entry(self.master, textvariable=self.iv, width=50).grid(row=1, column=1, pady=10)
tk.Label(self.master, text="明文(需填充至16字节的整数倍):").grid(row=2, column=0, pady=10)
tk.Entry(self.master, textvariable=self.plaintext, width=50).grid(row=2, column=1, pady=10)
tk.Label(self.master, text="密文:").grid(row=3, column=0, pady=10)
tk.Entry(self.master, textvariable=self.ciphertext, width=50).grid(row=3, column=1, pady=10)
# 创建加密和解密按钮
tk.Button(self.master, text="加密", command=self.encrypt).grid(row=4, column=0, pady=10)
tk.Button(self.master, text="解密", command=self.decrypt).grid(row=4, column=1, pady=10)
def encrypt(self):
# 获取输入的密钥、初始向量和明文
key = self.key.get()
iv = self.iv.get()
plaintext = self.plaintext.get()
# 将密钥和初始向量转换为字节类型
key = key.encode("utf-8")
iv = iv.encode("utf-8")
# 创建AES加密对象
cipher = AES.new(key, AES.MODE_CBC, iv)
# 将明文填充为16字节的整数倍
plaintext = pad(plaintext.encode("utf-8"), AES.block_size)
# 加密明文
ciphertext = cipher.encrypt(plaintext)
# 将密文转换为16进制字符串
ciphertext_hex = ciphertext.hex()
# 在界面上显示密文
self.ciphertext.set(ciphertext_hex)
def decrypt(self):
# 获取输入的密钥、初始向量和密文
key = self.key.get()
iv = self.iv.get()
ciphertext_hex = self.ciphertext.get()
# 将密钥和初始向量转换为字节类型
key = key.encode("utf-8")
iv = iv.encode("utf-8")
# 创建AES解密对象
cipher = AES.new(key, AES.MODE_CBC, iv)
# 将密文转换为字节类型
ciphertext = bytes.fromhex(ciphertext_hex)
# 解密密文
plaintext = cipher.decrypt(ciphertext)
# 去除填充
plaintext = plaintext.decode("utf-8").rstrip("\x00")
# 在界面上显示明文
self.plaintext.set(plaintext)
root = tk.Tk()
app = CBC_GUI(root)
root.mainloop()
```
以上代码实现了一个基于AES算法的CBC工作模式的可视化演示程序。通过输入密钥、初始向量和明文,可以进行加密和解密操作,同时在界面上显示密文和明文。程序使用了Python的tkinter模块来创建GUI界面,并使用了Crypto模块中的AES模块来进行加密和解密操作。
相关推荐
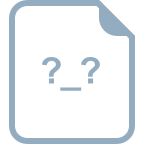
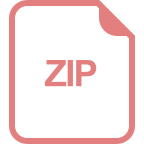
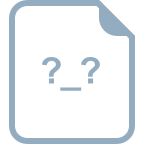
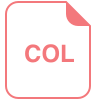
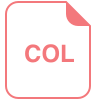
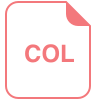
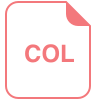
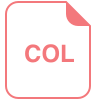









