httpurlconnection如何发送post请求
时间: 2023-02-20 15:46:32 浏览: 61
使用HttpURLConnection发送post请求非常简单,首先需要构建一个HttpURLConnection对象,然后设置请求方式,添加请求头,添加post参数,最后使用connect()方法发送请求,使用getInputStream()方法获取服务器的响应结果。
相关问题
httpurlconnection发送post请求头
下面是使用HttpURLConnection发送POST请求的示例代码:
```
try {
URL url = new URL("http://example.com/api");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("POST");
conn.setRequestProperty("Content-Type", "application/json; charset=UTF-8");
conn.setRequestProperty("Accept", "application/json");
conn.setDoOutput(true);
String input = "{\"username\":\"testuser\",\"password\":\"testpass\"}";
OutputStream os = conn.getOutputStream();
os.write(input.getBytes("UTF-8"));
os.flush();
BufferedReader br = new BufferedReader(new InputStreamReader((conn.getInputStream())));
String output;
while ((output = br.readLine()) != null) {
System.out.println(output);
}
conn.disconnect();
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
```
在这个示例中,我们创建一个URL对象,然后使用URL.openConnection()方法打开一个HttpURLConnection连接。我们设置请求方法为POST,并设置Content-Type和Accept请求头。然后,我们调用setDoOutput(true)方法,表示我们要向服务器发送数据。我们将数据写入OutputStream中,并调用flush()方法确保所有数据都被发送。最后,我们读取服务器的响应,并输出到控制台上。最后,我们断开连接。
HttpURLConnection 发送post请求出错 postman发送post请求没错
可能是因为你在使用 HttpURLConnection 发送 post 请求时没有正确设置请求头和请求参数。以下是一个简单的示例代码,你可以参考一下:
```java
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
public class HttpURLConnectionPostExample {
private static final String POST_URL = "http://example.com/api/post";
public static void main(String[] args) throws IOException {
URL url = new URL(POST_URL);
HttpURLConnection con = (HttpURLConnection) url.openConnection();
con.setRequestMethod("POST");
con.setRequestProperty("Content-Type", "application/json");
con.setDoOutput(true);
String requestBody = "{\"key1\":\"value1\",\"key2\":\"value2\"}";
OutputStream os = con.getOutputStream();
os.write(requestBody.getBytes());
os.flush();
os.close();
int responseCode = con.getResponseCode();
System.out.println("Response Code : " + responseCode);
BufferedReader in = new BufferedReader(new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
}
}
```
在上述代码中,我们首先创建了一个 `URL` 对象,表示要发送 post 请求的地址。然后我们使用 `HttpURLConnection` 类的 `openConnection()` 方法打开连接,并设置请求方法为 POST,设置请求头为 `Content-Type: application/json`,并将 `doOutput` 属性设置为 `true`,以便我们可以向服务器发送请求体。
接下来,我们将请求体写入输出流,并调用 `flush()` 和 `close()` 方法将其发送到服务器。然后我们使用 `getResponseCode()` 方法获取服务器响应的状态码,并使用 `getInputStream()` 方法获取服务器响应的输入流。最后,我们从输入流读取响应体,并将其转换为字符串。
相关推荐
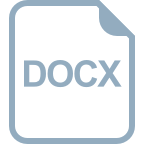
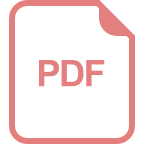
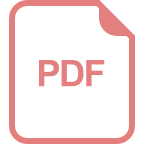












