用HTML+CSS写一个聊天气泡框
时间: 2024-03-15 16:21:05 浏览: 282
好的,以下是一个简单的聊天气泡框的HTML CSS代码:
HTML代码:
```
<div class="chat-bubble">
<p>Hello, how are you?</p>
</div>
```
CSS代码:
```
.chat-bubble {
background-color: #F5F5F5;
border-radius: 10px;
padding: 10px;
max-width: 70%;
margin: 10px;
position: relative;
}
.chat-bubble::before {
content: "";
position: absolute;
border-style: solid;
border-width: 10px 10px 10px 0;
border-color: transparent #F5F5F5 transparent transparent;
top: 50%;
transform: translateY(-50%);
left: -10px;
}
```
这个代码将创建一个灰色的聊天气泡框,它在左侧有一个三角形指示器。可以通过调整CSS属性来定制气泡框的样式,例如,更改背景颜色、边框半径、最大宽度等。
相关问题
用HTML+CSS+JS写一个聊天气泡框
好的,以下是一个简单的聊天气泡框示例,使用HTML、CSS和JavaScript:
HTML:
```html
<div class="chat-container">
<div class="chat-bubble outgoing">
<p>Hello!</p>
</div>
<div class="chat-bubble incoming">
<p>Hi there!</p>
</div>
</div>
```
CSS:
```css
.chat-container {
display: flex;
flex-direction: column;
align-items: flex-start;
justify-content: flex-start;
height: 400px;
padding: 20px;
overflow-y: scroll;
}
.chat-bubble {
display: inline-block;
max-width: 200px;
margin: 10px;
padding: 10px;
border-radius: 20px;
font-size: 16px;
line-height: 1.4;
}
.outgoing {
background-color: #0084ff;
color: #fff;
align-self: flex-end;
}
.incoming {
background-color: #f1f0f0;
color: #000;
align-self: flex-start;
}
```
JavaScript:
```javascript
// 获取聊天框容器元素
const chatContainer = document.querySelector('.chat-container');
// 添加新的聊天气泡
function addChatBubble(message, outgoing) {
const bubble = document.createElement('div');
bubble.classList.add('chat-bubble');
bubble.classList.add(outgoing ? 'outgoing' : 'incoming');
bubble.innerHTML = `<p>${message}</p>`;
chatContainer.appendChild(bubble);
}
// 测试
addChatBubble('Hello!', true);
addChatBubble('Hi there!', false);
```
这个示例创建了一个简单的聊天气泡框,使用了flex布局和圆角边框来实现。addChatBubble函数用于添加新的聊天气泡,可以根据需要设置出站消息或入站消息。
用HTML+CSS+vue写一个聊天气泡框
好的,以下是一个简单的聊天气泡框的实现方法:
HTML部分:
```html
<div id="chat-container">
<div class="message received">
<div class="message-content">
<p>Hello!</p>
</div>
</div>
<div class="message sent">
<div class="message-content">
<p>Hi there!</p>
</div>
</div>
</div>
```
CSS部分:
```css
#chat-container {
display: flex;
flex-direction: column;
}
.message {
max-width: 70%;
margin: 10px;
padding: 10px;
border-radius: 10px;
box-shadow: 0 2px 5px rgba(0, 0, 0, 0.3);
}
.received {
background-color: #eee;
align-self: flex-start;
}
.sent {
background-color: #0084ff;
color: #fff;
align-self: flex-end;
}
.message-content {
font-size: 16px;
line-height: 1.5;
padding: 5px;
}
```
Vue部分:
```vue
<template>
<div id="chat-container">
<div v-for="(message, index) in messages" :key="index" :class="message.type">
<div class="message-content">
<p>{{ message.content }}</p>
</div>
</div>
</div>
</template>
<script>
export default {
data() {
return {
messages: [
{ content: 'Hello!', type: 'received' },
{ content: 'Hi there!', type: 'sent' }
]
}
}
}
</script>
<style>
#chat-container {
display: flex;
flex-direction: column;
}
.message {
max-width: 70%;
margin: 10px;
padding: 10px;
border-radius: 10px;
box-shadow: 0 2px 5px rgba(0, 0, 0, 0.3);
}
.received {
background-color: #eee;
align-self: flex-start;
}
.sent {
background-color: #0084ff;
color: #fff;
align-self: flex-end;
}
.message-content {
font-size: 16px;
line-height: 1.5;
padding: 5px;
}
</style>
```
这是一个简单的聊天气泡框的实现方法,你可以根据实际需求进行修改和优化。
阅读全文
相关推荐
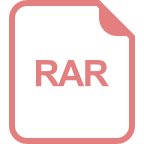
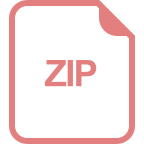
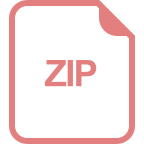












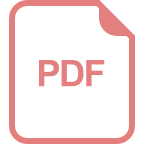
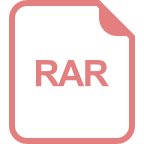