msp430g2553软件实现uart
时间: 2023-09-16 18:13:06 浏览: 70
要在MSP430G2553上实现UART通信,需要以下步骤:
1. 配置UART引脚
首先,需要将MSP430G2553的GPIO引脚配置为UART引脚。MSP430G2553有两个UART模块,分别为USCI_A0和USCI_B0。以USCI_A0为例,需要将P1.1和P1.2引脚配置为UART的RX和TX引脚。具体的配置方法可以参考MSP430G2553的数据手册。
2. 配置UART参数
在MSP430G2553上实现UART通信,还需要配置UART的参数,包括波特率、数据位、停止位和校验位等。可以使用MSP430G2553的硬件UART模块来实现这些配置。
3. 编写UART通信代码
最后,需要编写UART通信代码来实现数据的发送和接收。可以使用MSP430G2553的UART中断来实现数据的接收和发送。具体的实现方法可以参考MSP430G2553的数据手册和编程手册。
以下是一个简单的示例代码,用于在MSP430G2553上实现UART通信:
```c
#include <msp430.h>
#define UART_RX_BUF_SIZE 16
#define UART_TX_BUF_SIZE 16
unsigned char uart_rx_buf[UART_RX_BUF_SIZE];
unsigned char uart_tx_buf[UART_TX_BUF_SIZE];
unsigned char uart_rx_index = 0;
unsigned char uart_tx_index = 0;
unsigned char uart_rx_flag = 0;
void uart_init()
{
// 配置P1.1和P1.2为UART引脚
P1SEL |= BIT1 + BIT2;
P1SEL2 |= BIT1 + BIT2;
// 配置UART参数
UCA0CTL1 |= UCSSEL_2;
UCA0BR0 = 104;
UCA0BR1 = 0;
UCA0MCTL = UCBRS0;
// 使能UART接收中断
IE2 |= UCA0RXIE;
}
void uart_send(unsigned char *data, unsigned char len)
{
unsigned char i;
for (i = 0; i < len; i++)
{
while (!(IFG2 & UCA0TXIFG));
UCA0TXBUF = data[i];
}
}
#pragma vector=USCIAB0RX_VECTOR
__interrupt void uart_rx_isr()
{
unsigned char c = UCA0RXBUF;
if (uart_rx_index < UART_RX_BUF_SIZE)
{
uart_rx_buf[uart_rx_index++] = c;
}
if (c == '\n')
{
uart_rx_flag = 1;
uart_rx_index = 0;
}
}
int main(void)
{
WDTCTL = WDTPW + WDTHOLD; // 停止看门狗
uart_init();
__enable_interrupt();
while (1)
{
if (uart_rx_flag)
{
uart_send(uart_rx_buf, uart_rx_index);
uart_rx_flag = 0;
}
}
}
```
该代码实现了在MSP430G2553上通过UART接收数据,并将接收到的数据通过UART发送回去。具体实现方法请参考代码注释。
相关推荐
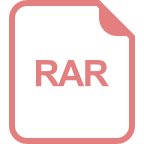














