python提取符串中的字符串
时间: 2024-09-12 17:07:26 浏览: 56
在Python中,提取字符串中的特定子串可以通过多种方法实现。这里介绍几种常用的方法:
1. 索引(Indexing):
使用索引可以直接访问字符串中的特定字符。Python字符串的索引是从0开始的,可以使用正索引也可以使用负索引。
```python
s = "Hello, World!"
print(s[7]) # 输出: W
print(s[-1]) # 输出: !
```
2. 切片(Slicing):
切片操作可以提取字符串的一部分。切片的语法是`[start:end]`,其中`start`是切片开始的位置索引,`end`是切片结束的位置索引,但不包括这个索引位置的字符。
```python
s = "Hello, World!"
print(s[7:9]) # 输出: Wo
print(s[:5]) # 输出: Hello
print(s[7:]) # 输出: World!
```
3. find() 方法:
`find()`方法用于查找字符串中子串的索引位置,如果找到了子串,则返回第一个字符的索引,如果没有找到,则返回-1。
```python
s = "Hello, World!"
print(s.find("World")) # 输出: 7
```
4. split() 方法:
`split()`方法通过指定分隔符对字符串进行切分,并返回一个包含子串的列表。如果不指定分隔符,默认是以空白字符(如空格、换行等)作为分隔符。
```python
s = "Hello, World! This is Python."
words = s.split()
print(words) # 输出: ['Hello,', 'World!', 'This', 'is', 'Python.']
```
5. 正则表达式(Regular Expressions):
使用Python的`re`模块,可以利用正则表达式灵活地查找和提取字符串中的模式。
```python
import re
s = "Hello, World! This is Python."
matches = re.findall("(\w+)", s)
print(matches) # 输出: ['Hello', 'World', 'This', 'is', 'Python']
```
阅读全文
相关推荐
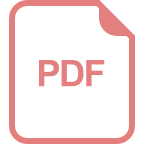
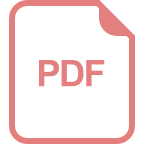
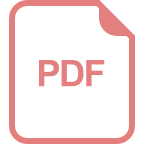


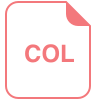











