beautifulsoup中如何获取固定id的div
时间: 2024-10-05 19:02:48 浏览: 19
在BeautifulSoup库中,你可以通过其强大的HTML解析功能来获取具有特定ID的`<div>`元素。下面是一个简单的步骤说明:
首先,你需要导入必要的模块:
```python
from bs4 import BeautifulSoup
import requests
```
然后,假设你想从一个网页中抓取某个ID名为"myDivId"的`<div>`元素,可以这样做:
```python
# 获取网页内容
url = "http://example.com" # 替换为你想要抓取的网页URL
response = requests.get(url)
html_content = response.text
# 使用BeautifulSoup解析HTML
soup = BeautifulSoup(html_content, 'html.parser')
# 定义要查找的ID
div_id = "myDivId"
# 找到指定ID的div元素
target_div = soup.find('div', id=div_id)
# 现在,target_div变量将包含找到的div元素
if target_div:
print(target_div.text) # 输出div的内容,如果有的话
else:
print(f"No div with ID '{div_id}' found.")
```
在这个例子中,`find()`方法接收两个参数,第一个参数是标签名,第二个参数是一个字典,其中键是属性(如'id'),值是要匹配的属性值。
相关问题
爬虫怎么只用requests中的Session对象获取登录后页面中的账号名
在使用Python的requests库的Session对象抓取登录后的页面时,由于Session可以保持登录状态,你可以直接像访问普通网页一样获取登录后的数据,包括账号名。假设你的登录页面POST表单字段是固定的,我们可以这样做:
首先,你需要模拟登录过程,创建一个Session对象并设置登录相关的Cookie或headers。这里是一个简单的示例:
```python
import requests
def login(username, password, base_url):
session = requests.Session()
login_data = {
'username': username,
'password': password,
# 假设这是登录页面的表单字段名,替换为实际的
}
# 发送登录请求
login_response = session.post(base_url + '/login', data=login_data)
# 检查登录状态,通常看状态码200或者检查是否有特定的验证信息
if login_response.status_code == 200 and 'logged_in' in login_response.text: # 这里只是示例,根据实际情况修改判断条件
return session # 成功登录,返回Session对象
else:
print("Login failed")
return None
# 使用登录后的Session获取账号名
def get_account_name(session, account_name_url):
response = session.get(account_name_url) # 发送GET请求获取用户信息页
account_name_html = response.text # 获取响应内容
# 假设账号名在某个标签内,如<div id="account-name">...</div>
account_name_selector = '#account-name' # CSS选择器,替换为实际的选择器
soup = BeautifulSoup(account_name_html, 'lxml') # 解析HTML
account_name = soup.select_one(account_name_selector).text.strip()
return account_name
# 使用示例
base_url = "https://www.example.com/"
username = "your_username"
password = "your_password"
session = login(username, password, base_url)
if session:
account_name = get_account_name(session, base_url + "/profile") # 替换为实际的用户信息页面URL
if account_name:
print(f"Account name: {account_name}")
else:
print("Could not find account name on the page.")
else:
print("Failed to log in.")
```
在这个例子中,我们先登录,然后通过`get_account_name`函数获取登录后的用户信息页面,并从中提取账号名。请注意,实际的CSS选择器和验证条件需要根据你要抓取的具体网页结构进行调整。
使用urllib库爬取当当网,用正则表达式获取产品名称和价格
使用Python的`urllib`库配合`re`(正则表达式)模块爬取网站信息是一个常见的任务。首先,你需要安装`requests`库以处理HTTP请求,然后你可以按照以下步骤进行操作:
1. 导入所需的库:
```python
import requests
import re
from bs4 import BeautifulSoup # 用于解析HTML
```
2. 发送GET请求到当当网首页,并获取响应内容:
```python
url = 'https://book.dangdang.com/' # 需要替换为你想爬取的具体页面URL
response = requests.get(url)
html_content = response.text
```
3. 解析HTML内容,通常会使用BeautifulSoup库解析页面结构:
```python
soup = BeautifulSoup(html_content, 'lxml')
```
4. 查找包含商品信息的部分。这一步可能需要查看网页源代码或开发者工具,找到产品名称和价格所在的标签及其类名、ID等属性。假设我们找到了一个包含所有商品列表的`div`元素,其类名为`product-list`:
```python
product_list = soup.find('div', class_='product-list')
```
5. 遍历每个商品项,查找名称和价格。这里再次假设名称在一个叫做`title`的子标签内,价格在`price`标签内。用正则表达式匹配价格(假设价格格式固定):
```python
def extract_product_info(product_item):
name_tag = product_item.find('span', class_='title') # 可能有不同的class名
if name_tag:
product_name = name_tag.text.strip()
price_tag = product_item.find('span', class_='price') # 类似地查找价格标签
if price_tag:
regex = r'\d+.\d+' # 正则表达式匹配浮点数的价格
try:
product_price = re.search(regex, price_tag.text).group()
except AttributeError:
product_price = None
return product_name, product_price
product_items = product_list.find_all('li') # 获取所有商品项
products = [extract_product_info(item) for item in product_items]
```
6. `products`变量将包含一个列表,其中每个元素都是一个元组,包含了对应产品的名称和价格。
**注意事项**:实际操作时,网站可能会有反爬虫机制或者动态加载数据,这时可能需要考虑使用Selenium等工具。同时,频繁爬取可能会触发网站的访问限制,请遵守网站的robots.txt规则并尊重版权。
阅读全文
相关推荐
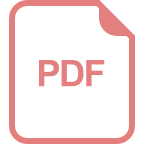
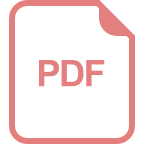
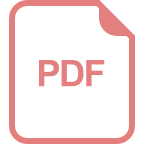
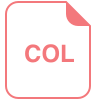
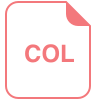
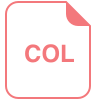
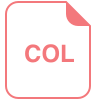
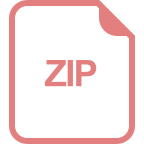
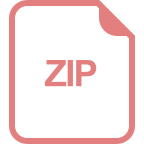
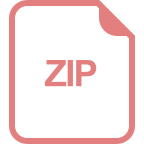
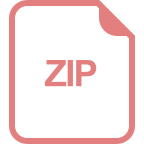