unity UnityWebRequest 上传文件添加时间字段和文件名字段 C# HttpListener接受
时间: 2024-01-14 10:02:55 浏览: 230
要在 Unity 中使用 UnityWebRequest 上传带有时间字段和文件名字段的文件,您可以使用以下代码:
```csharp
IEnumerator UploadFile(string url, string filePath, string fileName)
{
// Create a new UnityWebRequest
UnityWebRequest request = UnityWebRequest.Post(url, new List<IMultipartFormSection>());
// Set the content type
request.SetRequestHeader("Content-Type", "multipart/form-data");
// Create a new MultipartFormDataSection for the file
byte[] fileData = File.ReadAllBytes(filePath);
MultipartFormDataSection fileSection = new MultipartFormDataSection("file", fileData, fileName, "application/octet-stream");
// Add the file section to the request
request.multipartFormData.Add(fileSection);
// Add the time field to the request
request.AddField("time", DateTime.Now.ToString("yyyy-MM-dd HH:mm:ss"));
// Set the upload handler
request.uploadHandler = new UploadHandlerRaw(request.multipartFormData.CreateByteArray());
// Set the download handler
request.downloadHandler = new DownloadHandlerBuffer();
// Send the request
yield return request.SendWebRequest();
// Check for errors
if (request.result != UnityWebRequest.Result.Success)
{
Debug.LogError(request.error);
}
else
{
Debug.Log("File uploaded successfully");
}
}
```
要在 C# 中使用 HttpListener 接收带有时间字段和文件名字段的文件,您可以使用以下代码:
```csharp
HttpListener listener = new HttpListener();
listener.Prefixes.Add("http://localhost:8080/");
listener.Start();
while (true)
{
HttpListenerContext context = listener.GetContext();
if (context.Request.HttpMethod == "POST")
{
// Get the time field value
string time = context.Request.Form["time"];
// Get the file field values
HttpFileCollection files = context.Request.Files;
HttpPostedFile file = files[0];
string fileName = file.FileName;
Stream fileStream = file.InputStream;
// Save the file
using (FileStream output = new FileStream(fileName, FileMode.Create))
{
fileStream.CopyTo(output);
}
// Send a response
context.Response.StatusCode = 200;
context.Response.Close();
}
}
```
请注意,这只是一个基本示例代码,您需要根据您的具体需求进行修改。
阅读全文
相关推荐
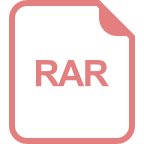
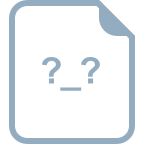
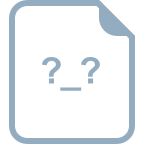
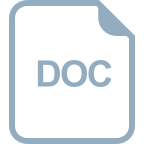




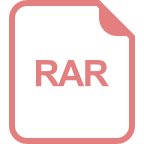
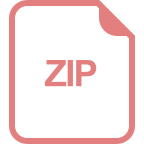
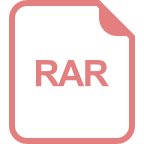
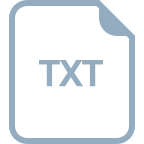
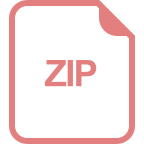