使用python写出可以识别图片中图形形状的代码
时间: 2023-06-11 11:05:56 浏览: 81
以下是一个使用Python和OpenCV库实现图形形状检测的例子代码:
```python
import cv2
# 读取图像
img = cv2.imread('shapes.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 二值化图像
_, thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)
# 查找轮廓
contours, _ = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 循环遍历每个轮廓
for cnt in contours:
# 获取轮廓近似多边形
approx = cv2.approxPolyDP(cnt, 0.01 * cv2.arcLength(cnt, True), True)
# 判断多边形是几边形
if len(approx) == 3:
shape = "Triangle"
elif len(approx) == 4:
x, y, w, h = cv2.boundingRect(cnt)
aspectRatio = float(w) / h
if aspectRatio >= 0.95 and aspectRatio <= 1.05:
shape = "Square"
else:
shape = "Rectangle"
elif len(approx) == 5:
shape = "Pentagon"
else:
shape = "Circle"
# 在图像中绘制图形名称
cv2.putText(img, shape, (cnt[0][0][0], cnt[0][0][1]), cv2.FONT_HERSHEY_SIMPLEX, 1, (0, 0, 0), 2)
# 显示图像
cv2.imshow('Image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
此代码假设输入图像名为"shapes.jpg",并且该图像包含三角形、矩形、正方形、五边形或圆形。运行代码后,它将检测每个形状并在图像中显示形状名称。
相关推荐
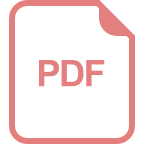
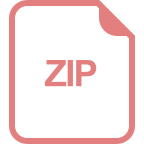
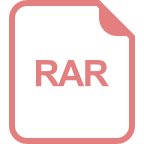














