1、编写一个程序,实现从键盘输入年份和月份,并输出该年、该月的天数
时间: 2023-09-09 21:05:00 浏览: 434
### 回答1:
我可以提供一个简单的程序供你参考:def days_in_month(year, month):
if month == 1 or month == 3 or month == 5 or month == 7 or month == 8 or month == 10 or month == 12:
return 31
elif month == 4 or month == 6 or month == 9 or month == 11:
return 30
else:
if (year % 4 == 0 and year % 100 != 0) or (year % 400 == 0):
return 29
else:
return 28year = int(input("请输入年份:"))
month = int(input("请输入月份:"))days = days_in_month(year, month)print(str(year) + "年" + str(month) + "月共有" + str(days) + "天。")
### 回答2:
要实现从键盘输入年份和月份,并输出该年、该月的天数,可以使用Python编写一个简单的程序:
```python
year = int(input("请输入年份:"))
month = int(input("请输入月份:"))
# 判断是否为闰年
if (year % 4 == 0 and year % 100 != 0) or (year % 400 == 0):
leap_year = True
else:
leap_year = False
# 判断每个月份的天数
if month in [1, 3, 5, 7, 8, 10, 12]:
days = 31
elif month in [4, 6, 9, 11]:
days = 30
elif month == 2:
if leap_year:
days = 29
else:
days = 28
else:
print("输入的月份无效!")
print(f"{year}年{month}月的天数为:{days}天")
```
该程序首先通过`input()`函数获取用户输入的年份和月份,然后使用`if...elif...else`语句判断该年份是否为闰年,根据月份的不同确定天数。最后输出结果。
### 回答3:
编写一个程序,实现从键盘输入年份和月份,并输出该年、该月的天数。具体步骤如下:
1. 首先,通过键盘输入获取年份和月份的值。
2. 判断年份是否为闰年。如果年份能被4整除但不能被100整除,或者能被400整除,那么该年份是闰年,否则是平年。
3. 根据月份和年份的判断结果,计算该月的天数。
- 如果月份为1、3、5、7、8、10、12,则该月的天数为31天。
- 如果月份为4、6、9、11,则该月的天数为30天。
- 如果月份为2,要判断是否为闰年。如果是闰年,则该月的天数为29天;如果是平年,则该月的天数为28天。
4. 输出该年、该月的天数。
下面是一个简单的示例代码:
```python
year = int(input("请输入年份:"))
month = int(input("请输入月份:"))
is_leap_year = False # 默认为平年
if year % 4 == 0 and year % 100 != 0 or year % 400 == 0:
is_leap_year = True
if month == 1 or month == 3 or month == 5 or month == 7 or month == 8 or month == 10 or month == 12:
days = 31
elif month == 4 or month == 6 or month == 9 or month == 11:
days = 30
elif month == 2:
if is_leap_year:
days = 29
else:
days = 28
else:
days = -1 # 输入的月份不合法
if days != -1:
print(f"{year}年{month}月的天数为{days}天。")
else:
print("输入的月份不合法。")
```
该程序可以根据用户输入的年份和月份,判断该年、该月的天数,并输出结果。
相关推荐
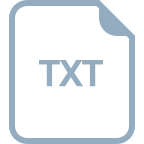














