python tkinter 编写记事本 打开py文件
时间: 2023-12-11 07:00:37 浏览: 166
使用Python中的Tkinter模块编写记事本的过程中,需要先打开一个py文件并将其保存为.py格式的Python脚本文件。接着,通过导入Tkinter模块并创建一个Tk对象作为记事本的窗口。然后,可以添加菜单栏、工具栏、文本编辑框等控件,并为它们添加相应的功能和事件处理。例如,可以在菜单栏中添加“打开文件”的选项,并为其绑定一个事件处理函数,使得用户点击该选项后可以选择并打开一个.py文件。在事件处理函数中,可以通过filedialog模块中的askopenfilename方法来获取用户选择的文件路径,并通过open方法将文件内容读取出来并显示在文本编辑框中。另外,还可以为记事本添加保存文件功能,使用户可以保存他们编辑过的文件。总之,使用Python的Tkinter模块编写记事本并打开.py文件,需要深入了解Tkinter的控件和事件处理机制,以及文件的读取和保存操作。通过这些知识和技能,我们就可以更加灵活和方便地实现记事本的功能和特性。
相关问题
用Python自行设计记事本或其他简单的界面设计
在Python中,可以使用多种库来设计简单的界面,比如tkinter、PyQt、PySide、wxPython等。这里我们以tkinter为例,它是一个标准的Python界面库,通常随Python一起安装。
以下是一个使用tkinter设计简单记事本的基本框架代码:
```python
import tkinter as tk
from tkinter import filedialog, messagebox
class SimpleNotepad:
def __init__(self, master):
self.master = master
master.title("简易记事本")
# 创建文本区域
self.text_area = tk.Text(master)
self.text_area.pack(fill=tk.BOTH, expand=1)
# 创建菜单栏
menubar = tk.Menu(master)
master.config(menu=menubar)
# 文件菜单
file_menu = tk.Menu(menubar, tearoff=0)
file_menu.add_command(label="新建", command=self.new_file)
file_menu.add_command(label="打开", command=self.open_file)
file_menu.add_command(label="保存", command=self.save_file)
file_menu.add_separator()
file_menu.add_command(label="退出", command=master.quit)
menubar.add_cascade(label="文件", menu=file_menu)
def new_file(self):
self.text_area.delete(1.0, tk.END)
def open_file(self):
filename = filedialog.askopenfilename()
if filename:
with open(filename, 'r') as file:
content = file.read()
self.text_area.delete(1.0, tk.END)
self.text_area.insert(1.0, content)
def save_file(self):
filename = filedialog.asksaveasfilename(defaultextension=".txt")
if filename:
with open(filename, 'w') as file:
content = self.text_area.get(1.0, tk.END)
file.write(content)
if __name__ == "__main__":
root = tk.Tk()
app = SimpleNotepad(root)
root.mainloop()
```
这段代码定义了一个`SimpleNotepad`类,它创建了一个包含文本编辑区域的窗口,并且具有文件菜单,可以执行新建、打开和保存文件的基本操作。
要运行这个记事本程序,你只需要将这段代码保存为`.py`文件,并确保你的系统中安装了Python环境,然后运行该文件即可。
用python给我做一个工具包包括小闹钟,计算器,自动关机,记事本,网站打开器,快捷键盘等
好的,以下是一个基于Python的工具包,其中包括小闹钟、计算器、自动关机、记事本、网站打开器、快捷键盘等功能。
```python
import time
import webbrowser
import os
from tkinter import *
from tkinter import messagebox
# 小闹钟
def alarm():
alarm_time = entry1.get()
while True:
time.sleep(1)
current_time = time.strftime("%H:%M:%S")
if current_time == alarm_time:
messagebox.showinfo("Alarm", "Time's up!")
break
# 计算器
def calculate():
expression = entry2.get()
try:
result = eval(expression)
messagebox.showinfo("Result", f"The result is {result}")
except:
messagebox.showerror("Error", "Invalid expression")
# 自动关机
def shutdown():
time = int(entry3.get())
os.system(f"shutdown /s /t {time}")
# 记事本
def notepad():
os.system("notepad")
# 网站打开器
def open_website():
website = entry5.get()
webbrowser.open(website)
# 快捷键盘
def shortcut():
root2 = Toplevel()
root2.title("Shortcuts")
root2.geometry("300x200")
label = Label(root2, text="Press Ctrl + C to copy")
label.pack(pady=10)
def copy():
root.clipboard_clear()
root.clipboard_append(entry6.get())
root.bind("<Control-c>", lambda x: copy())
# 创建GUI
root = Tk()
root.title("Toolbox")
root.geometry("400x500")
# 小闹钟
label1 = Label(root, text="Alarm Clock")
label1.pack(pady=10)
entry1 = Entry(root, width=10, font=("Helvetica", 20))
entry1.pack(pady=10)
button1 = Button(root, text="Set Alarm", command=alarm)
button1.pack(pady=10)
# 计算器
label2 = Label(root, text="Calculator")
label2.pack(pady=10)
entry2 = Entry(root, width=30, font=("Helvetica", 20))
entry2.pack(pady=10)
button2 = Button(root, text="Calculate", command=calculate)
button2.pack(pady=10)
# 自动关机
label3 = Label(root, text="Shutdown Timer (in secs)")
label3.pack(pady=10)
entry3 = Entry(root, width=10, font=("Helvetica", 20))
entry3.pack(pady=10)
button3 = Button(root, text="Shutdown", command=shutdown)
button3.pack(pady=10)
# 记事本
label4 = Label(root, text="Notepad")
label4.pack(pady=10)
button4 = Button(root, text="Open Notepad", command=notepad)
button4.pack(pady=10)
# 网站打开器
label5 = Label(root, text="Website Opener")
label5.pack(pady=10)
entry5 = Entry(root, width=30, font=("Helvetica", 20))
entry5.pack(pady=10)
button5 = Button(root, text="Open Website", command=open_website)
button5.pack(pady=10)
# 快捷键盘
label6 = Label(root, text="Shortcut Keyboard")
label6.pack(pady=10)
entry6 = Entry(root, width=30, font=("Helvetica", 20))
entry6.pack(pady=10)
button6 = Button(root, text="Open Shortcuts", command=shortcut)
button6.pack(pady=10)
root.mainloop()
```
你可以在控制台中运行这个程序,或者将代码保存到.py文件中,双击运行。
阅读全文
相关推荐
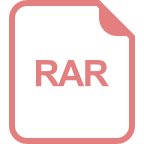
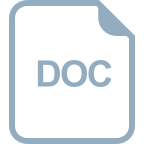
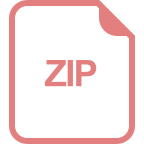
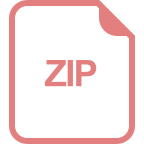
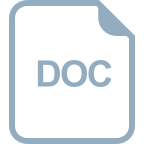
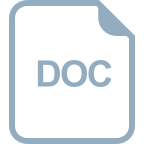
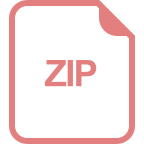
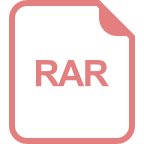
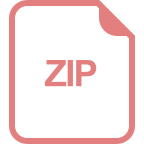
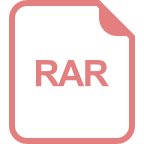
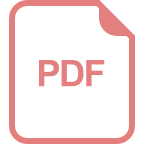
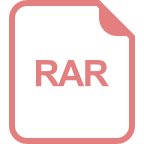
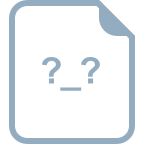
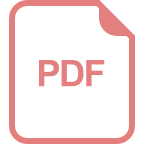
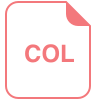