python+pyqt5 假如表单中有10个文本框与5个微调框,如何去快速判断这15个控件是不是全部填写有内容?
时间: 2024-09-22 10:09:22 浏览: 72
在Python中结合PyQt5,你可以通过遍历表单中的控件并检查它们的`text()`属性是否为空来判断所有文本框和微调框是否都有内容。这里是一个简单的示例:
```python
from PyQt5.QtWidgets import QApplication, QWidget, QLineEdit, QDoubleSpinBox
class Form(QWidget):
def __init__(self):
super().__init__()
self.init_ui()
def init_ui(self):
layout = QVBoxLayout()
# 创建10个文本框
for i in range(10):
text_box = QLineEdit()
text_box.setObjectName(f"text_{i}")
layout.addWidget(text_box)
# 创建5个微调框
for i in range(5):
spin_box = QDoubleSpinBox()
spin_box.setObjectName(f"spin_{i}")
layout.addWidget(spin_box)
self.setLayout(layout)
def check_filled(self):
filled = True
widgets = [getattr(self, obj_name) for obj_name in dir(self) if "text_" in obj_name or "spin_" in obj_name]
for widget in widgets:
if isinstance(widget, QLineEdit) and not widget.text():
filled = False
break
elif isinstance(widget, QDoubleSpinBox) and widget.value() == widget.minimum():
filled = False
break
return filled
# 使用示例
if __name__ == "__main__":
app = QApplication([])
form = Form()
form.show()
if form.check_filled():
print("所有控件都已填写")
else:
print("存在空的文本框或微调框")
app.exec_()
```
在这个例子中,我们首先初始化了一个窗口,并创建了10个文本框和5个微调框。然后,在`check_filled`函数中,我们获取所有名称中包含"text_"或"spin_"的对象,遍历它们并检查文本框是否有文本、微调框的值是否大于最小值(因为默认值可能是最小值)。如果有任何一个不符合条件,就将`filled`设置为False。
阅读全文
相关推荐
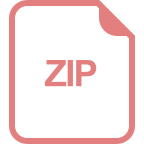
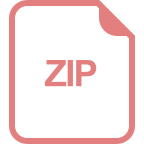
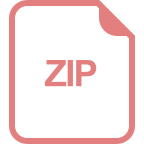
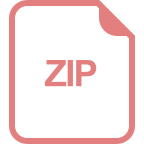
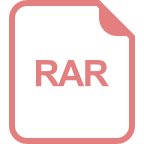
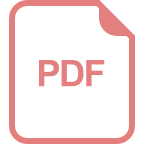
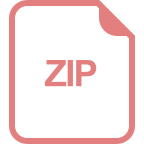
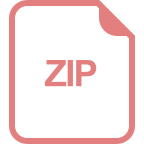
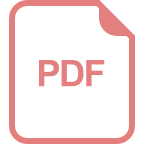
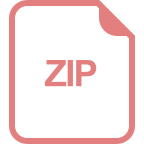
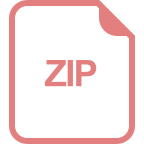
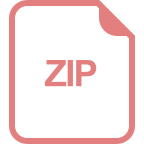
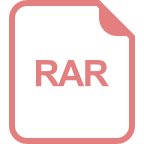
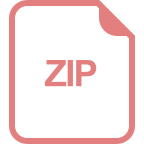
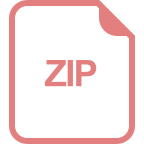
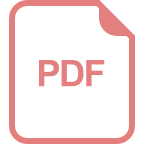
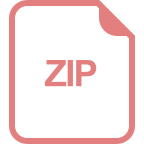