用python输入一段英文单词,输出重复字符不超过一半的
时间: 2024-11-03 07:16:48 浏览: 8
你可以使用Python编写一个简单的函数来实现这个功能。这里是一个基本的思路:
```python
def remove_half_duplicates(word):
word = list(word) # 转换为列表以便于操作
unique_chars = {} # 存储已见字符及其出现次数
for char in word:
if char not in unique_chars:
unique_chars[char] = 0
unique_chars[char] += 1 # 更新字符计数
half_unique_count = len(unique_chars) // 2 # 需要去除的一半独特字符的数量
new_word = [char for char, count in unique_chars.items() if count <= half_unique_count]
return ''.join(new_word)
# 示例用法
word = input("请输入一个英文单词:")
result = remove_half_duplicates(word)
print(f"去除了超过一半重复字符后的单词是:{result}")
相关问题
python输入一段英文求字符长度及包含的单词
你可以使用Python中的len()函数来获取字符串的长度,使用split()函数将字符串分割成单词,并使用len()函数获取单词个数。示例代码如下:
```python
# 获取字符串长度和单词列表
s = input("请输入一段英文:")
length = len(s)
words = s.split()
word_count = len(words)
# 输出结果
print("字符串长度为:", length)
print("包含的单词为:", words)
print("单词个数为:", word_count)
```
示例输出:
```
请输入一段英文:Hello world, this is a test.
字符串长度为: 28
包含的单词为: ['Hello', 'world,', 'this', 'is', 'a', 'test.']
单词个数为: 6
```
python输入一段英文统计单词个数
可以使用Python中的字符串和列表操作来实现统计英文单词个数的功能。
具体步骤如下:
1. 输入一段英文字符串,可以使用input()函数获取用户输入的字符串。
2. 将字符串转换为列表,可以使用split()函数将字符串按照空格分割成一个列表。
3. 遍历列表,统计单词个数。可以使用for循环遍历列表,使用len()函数获取列表长度,即单词个数。
4. 输出单词个数,可以使用print()函数输出单词个数。
下面是一个示例代码:
```
# 获取用户输入的英文字符串
text = input("请输入一段英文:")
# 将字符串转换为列表
words = text.split()
# 统计单词个数
count = len(words)
# 输出单词个数
print("单词个数为:", count)
```
运行代码后,输入一段英文字符串,程序会输出该字符串中单词的个数。
阅读全文
相关推荐
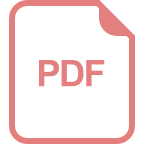
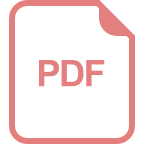
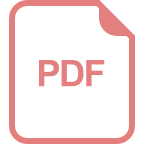













