六、编写函数实现利用链表对20个单词降序排列。
时间: 2024-10-13 21:03:47 浏览: 32
为了实现利用链表对20个单词进行降序排列,我们可以创建一个链表节点类`ListNode`,然后定义一个链表类`WordList`,包含插入单词的方法和一个排序功能。这里我会给出一个简化的Python示例:
```python
class ListNode:
def __init__(self, word):
self.word = word
self.next = None
class WordList:
def __init__(self):
self.head = None
# 插入单词到链表尾部
def insert_word(self, word):
if not self.head:
self.head = ListNode(word)
else:
current = self.head
while current.next:
current = current.next
current.next = ListNode(word)
# 升序比较两个单词并用于链表排序
def compare_words(self, node1, node2):
return -1 if node1.word > node2.word else (1 if node1.word < node2.word else 0)
# 使用归并排序算法降序排列链表
def sort_list_descending(self):
if not self.head or not self.head.next:
return
mid = self.partition(len(self.head)) # 分割链表
self.sort_list_descending(mid + 1) # 对右侧递归排序
self.sort_list_descending(1) # 对左侧递归排序
self.merge_sorted_lists() # 合并排序后的链表
def partition(self, size):
pivot = self.head
less = ListNode(None)
greater = ListNode(None)
less.next = pivot
for _ in range(size):
if self.compare_words(pivot.next, pivot) == -1:
pivot = pivot.next
temp = pivot.next
pivot.next = less.next
less.next = temp
return less.next
def merge_sorted_lists(self):
current1 = self.head
current2 = self.partition(len(self.head))
while current1 and current2:
if self.compare_words(current1.word, current2.word) >= 0:
current1 = current1.next
else:
current2.next = current1
current1 = current2
current2 = current2.next
if current1:
current2.next = current1
elif current2:
current1.next = current2
# 示例用法
word_list = WordList()
for i in range(1, 21): # 添加20个单词
word_list.insert_word(f"word_{i}")
word_list.sort_list_descending()
```
在这个例子中,我们首先插入单词,然后通过归并排序算法实现降序排列。请注意,这只是一个简化版本,实际应用可能需要考虑更多的边界条件和效率优化。
阅读全文
相关推荐
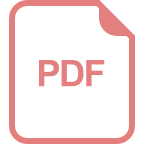
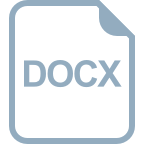
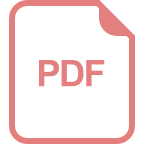
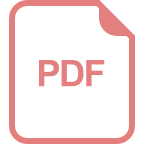
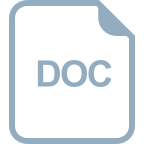
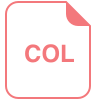
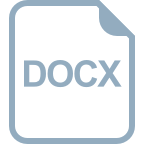
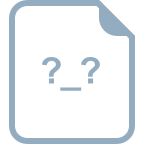
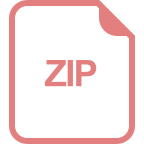
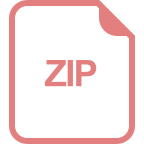