设计一个简单通讯录的C语言代码,它包含了基本的通讯录管理功能。
时间: 2024-12-17 14:37:51 浏览: 4
为了设计一个简单的通讯录管理程序,我们可以创建一个C语言程序,其中包含几个关键的功能模块:创建联系人、查看联系人列表、搜索特定联系人以及删除联系人。下面是一个简化的代码示例,使用文本文件作为数据存储媒介:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义联系人结构体
typedef struct {
char name[50];
char phone_number[20];
} Contact;
// 描述联系人数组
Contact contacts[100];
// 添加联系人到数组
void add_contact(char* name, char* phone_number) {
if (strlen(contacts) < 100) {
strcpy(contacts[strlen(contacts)], name);
strcpy(contacts[strlen(contacts) + 1], phone_number);
printf("Contact added.\n");
} else {
printf("Contact list is full.\n");
}
}
// 打印联系人列表
void view_contacts() {
for (int i = 0; i < strlen(contacts); i += 2) {
printf("%s | %s\n", contacts[i], contacts[i+1]);
}
}
// 搜索联系人
int search_contact(char* name) {
for (int i = 0; i < strlen(contacts); i += 2) {
if (strcmp(contacts[i], name) == 0) {
printf("%s | %s\n", contacts[i], contacts[i+1]);
return 1;
}
}
printf("Contact not found.\n");
return 0;
}
// 删除联系人
void delete_contact(char* name) {
for (int i = 0; i < strlen(contacts); i += 2) {
if (strcmp(contacts[i], name) == 0) {
memmove(&contacts[i], &contacts[i+2], (strlen(contacts) - i - 2) * sizeof(Contact));
printf("Contact deleted.\n");
break;
}
}
}
int main() {
char operation[] = "Enter operation (add, view, search, delete): ";
char name[50];
char phone_number[20];
while (1) {
printf(operation);
fgets(input, sizeof(input), stdin);
if (strcmp(input, "add") == 0) {
printf("Enter name: ");
fgets(name, sizeof(name), stdin);
name[strcspn(name, "\n")] = '\0'; // 清理换行符
printf("Enter phone number: ");
fgets(phone_number, sizeof(phone_number), stdin);
phone_number[strcspn(phone_number, "\n")] = '\0';
add_contact(name, phone_number);
} else if (strcmp(input, "view") == 0) {
view_contacts();
} else if (strcmp(input, "search") == 0) {
printf("Enter name to search: ");
fgets(name, sizeof(name), stdin);
name[strcspn(name, "\n")] = '\0';
search_contact(name);
} else if (strcmp(input, "delete") == 0) {
printf("Enter name to delete: ");
fgets(name, sizeof(name), stdin);
name[strcspn(name, "\n")] = '\0';
delete_contact(name);
} else {
printf("Invalid operation.\n");
}
printf("\n");
}
return 0;
}
```
阅读全文
相关推荐






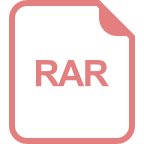









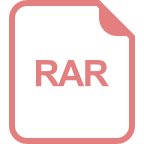

