用Android Studio开发一个记账本的代码
时间: 2023-11-03 12:26:06 浏览: 83
好的,下面是一个简单的记账本应用的代码示例,你可以参考一下:
AccountItem.java:
```java
public class AccountItem {
private String name;
private float amount;
public AccountItem(String name, float amount) {
this.name = name;
this.amount = amount;
}
public String getName() {
return name;
}
public float getAmount() {
return amount;
}
}
```
AccountAdapter.java:
```java
public class AccountAdapter extends BaseAdapter {
private List<AccountItem> accountList;
private Context context;
public AccountAdapter(Context context, List<AccountItem> accountList) {
this.context = context;
this.accountList = accountList;
}
@Override
public int getCount() {
return accountList.size();
}
@Override
public Object getItem(int position) {
return accountList.get(position);
}
@Override
public long getItemId(int position) {
return position;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
if (convertView == null) {
convertView = LayoutInflater.from(context).inflate(R.layout.item_account, parent, false);
}
TextView nameTv = convertView.findViewById(R.id.name_tv);
TextView amountTv = convertView.findViewById(R.id.amount_tv);
AccountItem item = accountList.get(position);
nameTv.setText(item.getName());
amountTv.setText(String.valueOf(item.getAmount()));
return convertView;
}
}
```
MainActivity.java:
```java
public class MainActivity extends AppCompatActivity {
private EditText nameEt;
private EditText amountEt;
private Button saveBtn;
private ListView accountLv;
private List<AccountItem> accountList;
private AccountAdapter accountAdapter;
private SharedPreferences sharedPreferences;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
nameEt = findViewById(R.id.name_et);
amountEt = findViewById(R.id.amount_et);
saveBtn = findViewById(R.id.save_btn);
accountLv = findViewById(R.id.account_lv);
accountList = new ArrayList<>();
accountAdapter = new AccountAdapter(this, accountList);
accountLv.setAdapter(accountAdapter);
sharedPreferences = getSharedPreferences("account_list", MODE_PRIVATE);
loadAccountList();
saveBtn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String name = nameEt.getText().toString();
float amount = Float.parseFloat(amountEt.getText().toString());
AccountItem item = new AccountItem(name, amount);
accountList.add(item);
accountAdapter.notifyDataSetChanged();
saveAccountList();
}
});
}
private void saveAccountList() {
SharedPreferences.Editor editor = sharedPreferences.edit();
Gson gson = new Gson();
String json = gson.toJson(accountList);
editor.putString("account_list", json);
editor.apply();
}
private void loadAccountList() {
Gson gson = new Gson();
String json = sharedPreferences.getString("account_list", "");
Type type = new TypeToken<List<AccountItem>>() {}.getType();
accountList = gson.fromJson(json, type);
if (accountList == null) {
accountList = new ArrayList<>();
}
accountAdapter = new AccountAdapter(this, accountList);
accountLv.setAdapter(accountAdapter);
}
@Override
protected void onStop() {
super.onStop();
saveAccountList();
}
}
```
上面的代码示例包含了一个 AccountItem 类,表示一个账单条目,一个 AccountAdapter 类,用于将账单信息显示在 ListView 中,以及一个 MainActivity 类,实现了记账本应用的主界面和核心逻辑。注意,在实现保存和读取账单信息时,我们使用了 SharedPreferences 来实现数据的持久化存储。
相关推荐
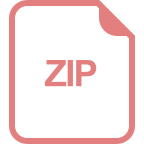
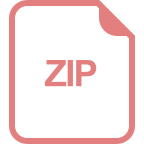
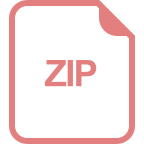
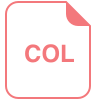
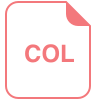
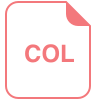
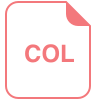
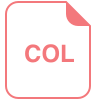









