linux下ioctl中,buff[10]; 应用下发指针buff,驱动中如何保存下应用空间buff地址,并打印出应用空间buff的地址
时间: 2024-05-10 15:17:27 浏览: 142
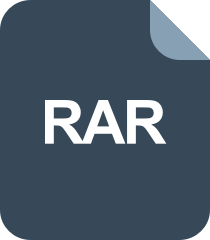
linux-lcd.rar_linux中lcd_linux应用
在驱动程序中,我们可以通过 `copy_from_user()` 或者 `copy_to_user()` 等函数来读写应用程序的缓冲区。当应用程序在调用 `ioctl()` 系统调用时,会将缓冲区的地址和长度作为参数传递给驱动程序。我们可以通过结构体 `file` 和 `ioctl` 命令号来获取这些参数。
以下是一个示例代码,展示了如何在驱动程序中保存应用程序缓冲区的地址,并打印出来:
```c
#include <linux/fs.h>
#include <linux/ioctl.h>
#include <linux/uaccess.h>
#define MY_IOCTL_CMD _IOR('q', 1, int)
static long my_ioctl(struct file *file, unsigned int cmd, unsigned long arg)
{
int *buff = NULL;
switch (cmd) {
case MY_IOCTL_CMD:
// 从用户空间复制数据到 kernel 空间
if (copy_from_user(&buff, (int __user *)arg, sizeof(int *)))
return -EFAULT;
// 打印缓冲区地址
printk(KERN_INFO "User space buffer address: %p\n", buff);
// ... do something with buffer ...
break;
default:
return -ENOTTY;
}
return 0;
}
```
在应用程序中,我们可以将缓冲区地址传递给 `ioctl()` 系统调用:
```c
int fd;
int *buff = malloc(10 * sizeof(int));
if (!buff)
return -ENOMEM;
fd = open("/dev/my_device", O_RDONLY);
if (fd < 0) {
free(buff);
return fd;
}
if (ioctl(fd, MY_IOCTL_CMD, &buff)) {
perror("ioctl");
close(fd);
free(buff);
return -1;
}
close(fd);
free(buff);
```
注意,我们在应用程序中传递的参数是 `&buff`,这是因为我们要传递指向缓冲区地址的指针的地址。在驱动程序中,我们需要使用 `copy_from_user()` 函数来将这个指针地址复制到内核空间中,在驱动程序中获取缓冲区地址的方式是通过 `int *buff = NULL;` 定义一个指针变量,然后将该指针变量赋值为从用户空间中复制过来的指针地址 `copy_from_user(&buff, (int __user *)arg, sizeof(int *)))`。最后,我们可以使用 `printk()` 函数来打印缓冲区的地址。
阅读全文
相关推荐
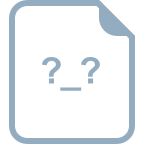
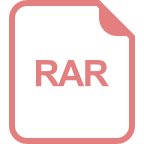
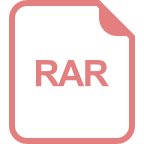