springboot整合OSS
时间: 2023-09-06 18:10:19 浏览: 67
Spring Boot可以很方便地集成阿里云的对象存储服务(OSS)。下面是整合步骤:
1. 首先,在你的Spring Boot项目中添加阿里云OSS的依赖,可以在pom.xml文件中加入以下代码:
```xml
<dependency>
<groupId>com.aliyun.oss</groupId>
<artifactId>aliyun-sdk-oss</artifactId>
<version>3.13.1</version>
</dependency>
```
2. 创建一个配置类,用于配置阿里云OSS的相关参数,比如accessKeyId、accessKeySecret等。可以在src/main/java目录下创建一个`OSSConfig.java`文件,并添加如下代码:
```java
import com.aliyun.oss.OSS;
import com.aliyun.oss.OSSClientBuilder;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class OSSConfig {
@Value("${oss.endpoint}")
private String endpoint;
@Value("${oss.accessKeyId}")
private String accessKeyId;
@Value("${oss.accessKeySecret}")
private String accessKeySecret;
@Bean
public OSS ossClient() {
return new OSSClientBuilder().build(endpoint, accessKeyId, accessKeySecret);
}
}
```
3. 在application.properties或application.yml文件中添加配置,例如:
application.properties:
```properties
oss.endpoint=your_endpoint
oss.accessKeyId=your_access_key_id
oss.accessKeySecret=your_access_key_secret
```
application.yml:
```yaml
oss:
endpoint: your_endpoint
accessKeyId: your_access_key_id
accessKeySecret: your_access_key_secret
```
4. 在你的代码中使用已经注入的OSS bean来操作对象存储服务。例如,上传文件:
```java
import com.aliyun.oss.OSS;
import com.aliyun.oss.model.PutObjectRequest;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class OSSService {
@Autowired
private OSS ossClient;
public void uploadFile(String bucketName, String objectName, String filePath) {
ossClient.putObject(new PutObjectRequest(bucketName, objectName, new File(filePath)));
}
}
```
这样就完成了Spring Boot与阿里云OSS的整合,你可以根据自己的需求进行更多操作,比如下载文件、删除文件等。希望对你有帮助!如果还有其他问题,请继续提问。
相关推荐
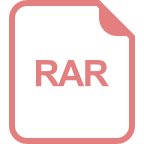














