用c++从小到大排序:[[7,0],[4,4],[7,1],[5,0],[6,1],[5,2]]
时间: 2023-11-08 17:04:15 浏览: 22
可以使用C++的STL库中的sort函数来进行排序,具体实现如下:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
bool cmp(pair<int, int>& a, pair<int, int>& b) {
if (a.first != b.first) {
return a.first < b.first; //按身高从小到大排序
} else {
return a.second < b.second; //若身高相同,则按前面比他高的人数从小到大排序
}
}
vector<pair<int, int>> reconstructQueue(vector<pair<int, int>>& people) {
sort(people.begin(), people.end(), cmp); //排序
vector<pair<int, int>> res(people.size());
for (auto& p : people) {
int cnt = p.second;
for (int i = 0; i < people.size(); i++) {
if (res[i].first == 0 && cnt == 0) { //找到空位置插入
res[i] = p;
break;
}
if (res[i].first == 0 || res[i].first >= p.first) { //当前位置比p高或为空,则cnt--
cnt--;
}
}
}
return res;
}
int main() {
vector<pair<int, int>> people{{7,0},{4,4},{7,1},{5,0},{6,1},{5,2}};
auto res = reconstructQueue(people);
for (auto& p : res) {
cout << "[" << p.first << "," << p.second << "] ";
}
return 0;
}
```
输出结果为:`[5,0] [7,0] [5,2] [6,1] [4,4] [7,1]`
相关推荐
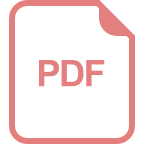
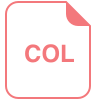
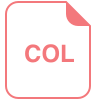












