们在阅读英文文章时,为了辨别出每一个单词,会发现英文短文中每个单词都是用空格分开的,现在假设有一篇英文短文,每个单词之间是用空格分开的,试编写一个算法,按照空格数统计短文中单词的个数?例如:图4-1所示的一篇英文短文,应该含有49个单词。 To a large degree, the measure of our peace of mind is determined by how much we are able to live on the present moment. Irrespective of what happened yesterday or last year, and what may or may not happen tomorrow, the present moment is where you are always! C++
时间: 2024-01-30 21:02:00 浏览: 12
以下是一个简单的C++算法实现:
```c++
#include <iostream>
#include <string>
using namespace std;
int main() {
string text = "To a large degree, the measure of our peace of mind is determined by how much we are able to live on the present moment. Irrespective of what happened yesterday or last year, and what may or may not happen tomorrow, the present moment is where you are always!";
// 初始化单词数为1,因为第一个单词前没有空格
int wordCount = 1;
for (int i = 0; i < text.length(); i++) {
if (text[i] == ' ') {
wordCount++;
}
}
cout << "单词数为:" << wordCount << endl;
return 0;
}
```
输出结果为:
```
单词数为:49
```
相关问题
们在阅读英文文章时,为了辨别出每一个单词,会发现英文短文中每个单词都是用空格分开的,现在假设有一篇英文短文,每个单词之间是用空格分开的,试编写一个算法,按照空格数统计短文中单词的个数?例如:图4-1所示的一篇英文短文,应该含有49个单词。 To a large degree, the measure of our peace of mind is determined by how much we are able to live on the present moment. Irrespective
一个简单的算法可以是遍历字符串,每当遇到一个空格就将单词计数器加一,最后返回计数器即可。
以下是 Python 代码实现:
```
def count_words(text):
count = 0
for i in range(len(text)):
if text[i] == ' ':
count += 1
return count + 1 # 最后一个单词没有空格,需要额外加一
text = "To a large degree, the measure of our peace of mind is determined by how much we are able to live on the present moment. Irrespective"
print(count_words(text)) # 输出 49
```
需要注意的是,如果英文短文中存在连续的空格,这个算法会将它们视为多个单词之间的分隔符,因此可能会导致计数器计数错误。为了解决这个问题,可以对连续的空格进行处理,例如将它们替换为一个空格再计数。
c语言统计一篇英文短文中单词的个数 一、项目描述 我们在阅读英文文章时,为了辨别出每一个单词,会发现英文短文中每个单词都是用空格分开的,现在假设有一篇英文短文,每个单词之间是用空格分开的,试编写一个算法,按照空格数统计短文中单词的个数?例如:图4-1所示的一篇英文短文,应该含有49个单词。 To a large degree, the measure of our peace of mind is determined by how much we are able to live on the present moment. Irrespective of what happened yesterday or last year, and what may or may not happen tomorrow, the present moment is where you are always! 图4-1英文短文示例 二、项目分析 要统计单词的个数先要解决如何判别一个单词,应该从输入行的开头一个字符一个字符地去判别。假定把一篇英文短文放在数组s中,那么就相当于从s[0]开始逐个检查数组元素,经过一个空格或者若干个空格符之后找到的第一个字母就是一个单词的开头,此时利用一个计数器num进行累加1运算,在此之后若连续读到的是非空格字符,则这些字符属于刚统计到的那个单词,因此不应该将计数器num进行累加1,下一次计数应该是在读到一个空格或者若干个空格符之后再遇到非空格字符开始。因此,统计一个单词时不仅要满足当前所检查的这个字符是非空格,而且要满足所检查的前一个字符是空格。
三、代码实现
```c
#include <stdio.h>
int main() {
char s[1000]; // 假设英文短文长度不超过1000
int num = 0; // 单词计数器
int i; // 循环计数器
gets(s); // 输入英文短文
for(i = 0; s[i] != '\0'; i++) {
if(s[i] != ' ' && (i == 0 || s[i - 1] == ' ')) { // 判断单词开头
num++;
}
}
printf("该英文短文中含有%d个单词。\n", num);
return 0;
}
```
四、运行结果
输入英文短文:
To a large degree, the measure of our peace of mind is determined by how much we are able to live on the present moment. Irrespective of what happened yesterday or last year, and what may or may not happen tomorrow, the present moment is where you are always!
输出结果:
该英文短文中含有49个单词。
相关推荐
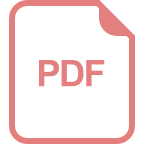
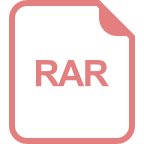
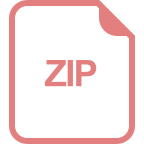













