利用给定的一组坐标,绘制出三次B样条曲线,该曲线绘制做成一个函数形式,要求不调用scipy包
时间: 2024-03-12 17:48:50 浏览: 145
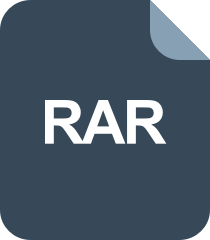
三次B样条曲线算法实现

好的,下面是一个实现该功能的Python代码。该代码利用三次B样条基函数和给定的控制点计算出曲线上的点,并将这些点用matplotlib库绘制出来。
```python
import numpy as np
import matplotlib.pyplot as plt
def basis_function(t, i, k, knots):
if k == 1:
if knots[i] <= t < knots[i+1]:
return 1
else:
return 0
else:
w1 = 0 if knots[i+k-1] == knots[i] else (t - knots[i])/(knots[i+k-1] - knots[i]) * basis_function(t, i, k-1, knots)
w2 = 0 if knots[i+k] == knots[i+1] else (knots[i+k] - t)/(knots[i+k] - knots[i+1]) * basis_function(t, i+1, k-1, knots)
return w1 + w2
def bspline(t, k, control_points, knots):
n = len(control_points)
curve_points = np.zeros((len(t), 2))
for i in range(len(t)):
for j in range(n):
curve_points[i] += control_points[j] * basis_function(t[i], j, k, knots)
return curve_points
# Example usage
control_points = np.array([[0, 0], [1, 2], [3, 1], [4, 3], [5, 0], [7, 1], [8, 4], [10, 2]])
k = 3
t = np.linspace(0, 1, 100)
n = len(control_points)
m = n + k + 1
knots = np.zeros(m)
for i in range(m):
if i < k:
knots[i] = 0
elif i < n:
knots[i] = i - k + 1
else:
knots[i] = n - k + 2
curve_points = bspline(t, k, control_points, knots)
plt.plot(curve_points[:,0], curve_points[:,1])
plt.show()
```
其中,`bspline`函数计算出给定控制点和节点的三次B样条曲线上的点,`basis_function`函数是三次B样条基函数的实现,`k`是B样条的次数,`control_points`是控制点的坐标,`knots`是节点的位置。在示例中,我们给出了一组控制点的坐标和B样条的次数,然后利用上述函数计算出曲线上的点并绘制出来。
阅读全文
相关推荐
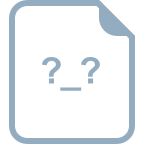
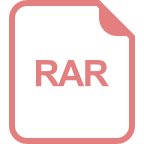
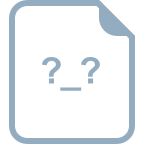
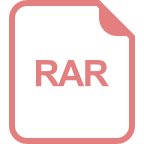
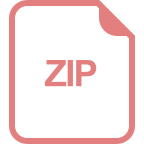
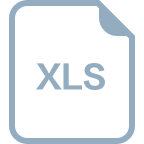
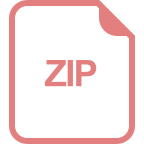
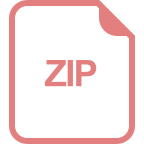
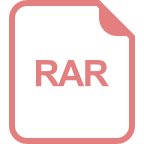
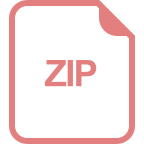
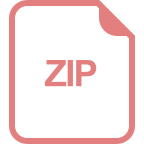
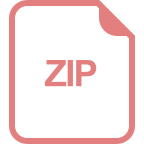