产生0-7之间的随机整数
时间: 2024-03-15 17:47:27 浏览: 19
可以使用`Math.random()`方法生成0到1之间的随机小数,并通过一些数学运算将其转换成0到7之间的随机整数。以下是一种实现方式:
```javascript
const randomInt = Math.floor(Math.random() * 8);
```
首先,`Math.random()`方法会生成一个0到1之间的随机小数,包括0但不包括1。然后,将这个随机小数乘以8,得到的结果是0到8之间的随机小数,包括0但不包括8。最后,使用`Math.floor()`方法将这个随机小数向下取整,得到0到7之间的随机整数。
相关问题
python产生10-20的随机整数
在Python中,可以用random模块中的randint函数生成10到20之间的随机整数。具体代码如下:
```
import random
num = random.randint(10, 20)
print(num)
```
运行多次可以看到每次输出的数值都是随机的且在10到20之间。
利用Random类产生5个20-30之间的随机整数
好的,以下是利用Random类产生5个20-30之间的随机整数的代码实现:
```java
import java.util.Random;
public class RandomDemo {
public static void main(String[] args) {
Random rand = new Random();
for (int i = 0; i < 5; i++) {
int num = rand.nextInt(11) + 20; // 生成20-30之间的随机整数
System.out.println(num);
}
}
}
```
运行程序后,会生成5个范围在20-30之间的随机整数并输出。在生成随机数时,需要注意的是nextInt方法的参数表示生成的随机数的上限,因此需要将上限设置为11,这样生成的随机数范围就在0-10之间,再加上20就得到20-30之间的随机整数了。
相关推荐
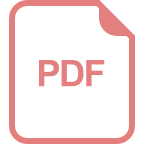












