pytorch导入模型并进行测试
时间: 2023-06-11 21:06:46 浏览: 73
导入模型并进行测试的步骤如下:
1. 安装PyTorch和相关依赖。
2. 加载模型。使用`torch.load()`函数加载已训练好的模型参数。
```python
import torch
# 加载模型
model = torch.load('model.pth')
```
3. 准备测试数据。根据模型输入数据的格式准备测试数据。
```python
# 准备测试数据
test_data = [
[0.1, 0.2, 0.3],
[0.4, 0.5, 0.6]
]
```
4. 将测试数据转换为PyTorch张量。
```python
# 转换为PyTorch张量
test_data_tensor = torch.tensor(test_data)
```
5. 将模型设置为评估模式。使用`model.eval()`函数将模型设置为评估模式。
```python
# 将模型设置为评估模式
model.eval()
```
6. 使用模型进行推断。将测试数据输入模型,得到模型的输出结果。
```python
# 使用模型进行推断
with torch.no_grad():
output = model(test_data_tensor)
```
7. 处理输出结果。根据模型输出的格式对结果进行进一步处理。
```python
# 处理输出结果
print(output)
```
完整的代码示例:
```python
import torch
# 加载模型
model = torch.load('model.pth')
# 准备测试数据
test_data = [
[0.1, 0.2, 0.3],
[0.4, 0.5, 0.6]
]
# 转换为PyTorch张量
test_data_tensor = torch.tensor(test_data)
# 将模型设置为评估模式
model.eval()
# 使用模型进行推断
with torch.no_grad():
output = model(test_data_tensor)
# 处理输出结果
print(output)
```
相关推荐
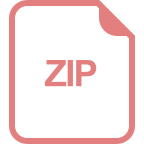
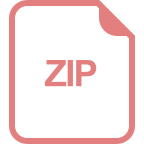
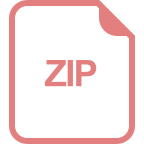














