el-uplaod中的base64如何解码
时间: 2024-05-06 21:21:10 浏览: 36
base64解码可以使用Python中的base64库进行实现,代码如下:
```python
import base64
encoded_string = '<base64 encoded string>' # 将base64编码的字符串替换成实际的字符串
decoded_string = base64.b64decode(encoded_string).decode('utf-8') # 解码并转换为utf-8编码的字符串
print(decoded_string)
```
请注意,在实际应用中,需要将`<base64 encoded string>`替换为真正的base64编码字符串。
相关问题
el-uplaod多文件上传
el-upload 组件可以实现多文件上传。你需要设置 `:multiple="true"` 属性,以允许用户选择多个文件。同时,使用 `:auto-upload="false"` 属性可以禁止自动上传,而是需要手动触发上传事件。
以下是一个示例代码:
```
<template>
<div>
<el-upload
action="your upload url"
:multiple="true"
:auto-upload="false"
:on-change="handleChange"
>
<el-button slot="trigger">选择文件</el-button>
<el-button style="margin-left: 10px;" type="primary" @click="handleUpload">上传文件</el-button>
</el-upload>
</div>
</template>
<script>
export default {
methods: {
handleChange(file, fileList) {
console.log(file, fileList);
},
handleUpload() {
const formData = new FormData();
// 将文件加入 formData
this.$refs.upload.uploadFiles(formData);
}
}
}
</script>
```
在 `handleUpload` 方法中,我们创建了一个 `FormData` 对象,并将选择的文件加入到 formData 中。然后,通过 `this.$refs.upload.uploadFiles(formData)` 方法手动触发上传事件。在 `handleChange` 方法中,我们可以监听文件的选择和上传状态变化,并进行相应的处理。
用代码实现u-uplaod获取选中的图片
可以使用以下代码实现:
```javascript
const input = document.createElement('input');
input.type = 'file';
input.accept = 'image/*';
input.onchange = (event) => {
const file = event.target.files[0];
const formData = new FormData();
formData.append('file', file);
fetch('https://api.ucloud.cn/file/upload', {
method: 'POST',
body: formData,
headers: {
'Authorization': 'UCloud ' + '<your public key>' + ':' + '<your signature>',
},
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
};
input.click();
```
其中,`<your public key>` 和 `<your signature>` 需要替换为你自己的 UCloud 公钥和签名。
相关推荐
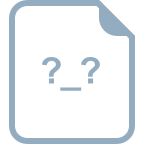



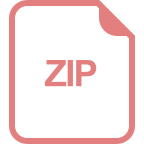
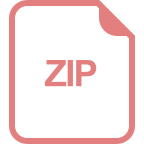
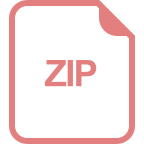