RF算法提取光谱数据特征波长python代码
时间: 2023-08-29 16:07:17 浏览: 108
我可以回答这个问题,以下是一个可以用RF算法提取光谱数据特征波长的Python代码示例:
```
from sklearn.ensemble import RandomForestRegressor
# 假设光谱数据存储在X数组中,特征波长存储在y数组中
rf = RandomForestRegressor(n_estimators=100, random_state=42)
rf.fit(X, y)
# 获取特征重要性
importances = rf.feature_importances_
# 将特征重要性从高到低排序
indices = np.argsort(importances)[::-1]
# 输出特征波长的重要性排名
for f in range(X.shape[1]):
print("%d. feature %d (%f)" % (f + 1, indices[f], importances[indices[f]]))
```
希望这能对你有所帮助!
相关问题
RF算法提取特征波长python代码
我可以回答这个问题。RF算法提取特征波长的Python代码可以使用scikit-learn中的RandomForestRegressor模块实现。以下是示例代码:
```python
from sklearn.ensemble import RandomForestRegressor
# X是特征矩阵,y是目标向量
rf = RandomForestRegressor()
rf.fit(X, y)
# 提取特征重要性
importances = rf.feature_importances_
```
其中X和y是数据集中的特征矩阵和目标向量,fit()方法用于训练模型,feature_importances_属性用于提取特征重要性。
用随机森林算法填充数据的python代码
当使用随机森林算法填充数据时,你可以使用 `sklearn.ensemble` 中的 `RandomForestRegressor` 或 `RandomForestClassifier` 类。下面是一个使用随机森林回归算法填充数据的示例代码:
```python
import numpy as np
from sklearn.ensemble import RandomForestRegressor
# 创建一个带有缺失值的数据集
X = np.array([[1, 2, np.nan],
[4, np.nan, 6],
[7, 8, 9],
[np.nan, 11, 12]])
# 将缺失值替换为0
X_filled = np.nan_to_num(X, nan=0)
# 创建一个随机森林回归模型
rf = RandomForestRegressor()
# 训练模型并预测缺失值
rf.fit(X_filled[:, :-1], X_filled[:, -1])
predicted_values = rf.predict(X_filled[:, :-1])
# 将预测值填充回数据集
X[:, -1] = np.where(np.isnan(X[:, -1]), predicted_values, X[:, -1])
print(X)
```
这段代码中,首先创建了一个带有缺失值的数据集 `X`。然后,使用 `np.nan_to_num` 将缺失值替换为0,以便随机森林模型可以处理。接下来,创建一个随机森林回归模型 `rf`,并用已填充的数据集训练模型。最后,使用训练好的模型预测缺失值,并将预测值填充回原始数据集 `X`。
相关推荐
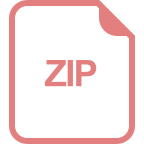
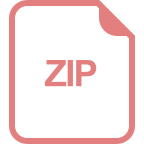
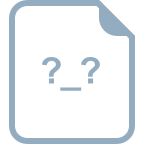
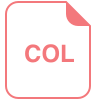











