如果是使用mybatis-plus的情况下,怎么在事务提交前,获取最新的数据库中的值。
时间: 2024-03-16 07:44:22 浏览: 15
在使用Mybatis-Plus的情况下,在事务提交前获取最新的数据库中的值可以通过以下步骤实现:
1. 在需要获取最新值的方法中,先查询数据库中的最新值并保存。例如:
```java
@Transactional
public void yourMethod(Long id) {
YourEntity entity = yourMapper.selectById(id);
if (entity == null) {
throw new RuntimeException("Entity not found");
}
YourEntity latestEntity = yourMapper.selectById(id); // 查询最新的实体类
// 在这里执行其他操作,例如修改实体类的属性值
yourMapper.updateById(entity); // 更新实体类到数据库
}
```
这里假设你的方法名为 `yourMethod`,需要更新的实体类主键为 `id`,并且使用了事务注解 `@Transactional`。在方法内部,先通过主键查询实体类,并保存在 `entity` 变量中。然后再次查询最新的实体类,并保存在 `latestEntity` 变量中。
2. 在事务提交前,可以使用 Spring 提供的 `TransactionSynchronizationManager` 类来注册一个 `TransactionSynchronization` 对象,在事务提交前执行一些操作。例如:
```java
@Transactional
public void yourMethod(Long id) {
YourEntity entity = yourMapper.selectById(id);
if (entity == null) {
throw new RuntimeException("Entity not found");
}
YourEntity latestEntity = yourMapper.selectById(id); // 查询最新的实体类
// 在这里执行其他操作,例如修改实体类的属性值
TransactionSynchronizationManager.registerSynchronization(new TransactionSynchronization() {
@Override
public void beforeCommit(boolean readOnly) {
entity.refresh(); // 刷新实体类的属性值
// 在这里使用 latestEntity 变量获取最新的实体类属性值
}
});
yourMapper.updateById(entity); // 更新实体类到数据库
}
```
在这里,我们注册了一个 `TransactionSynchronization` 对象,在事务提交前执行 `beforeCommit()` 方法。在该方法中,我们可以调用 `entity.refresh()` 方法刷新实体类的属性值,并使用 `latestEntity` 变量获取最新的实体类属性值。
这样,就可以在事务提交前获取最新的数据库中的值。注意,需要使用事务注解 `@Transactional` 来确保操作在一个事务中执行。
相关推荐
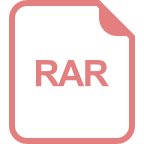
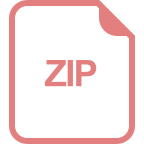
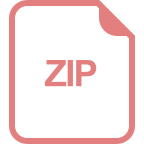














