unsupported operand type(s) for -: 'numpy.str_' and 'int'
时间: 2023-08-01 12:11:20 浏览: 119
这个错误通常出现在你尝试对一个字符串和一个整数执行减法操作时。Python不支持这种操作,因为它们是不兼容的数据类型。你需要确保你的操作是针对相同的数据类型。如果你想将字符串转换为整数来执行减法操作,可以使用int()函数将字符串转换为整数。例如,如果你有一个名为s的字符串,你可以使用int(s)将它转换为整数。
相关问题
TypeError: unsupported operand type(s) for -: 'numpy.str_' and 'float'
This error occurs when you try to perform a mathematical operation between a numpy string and a float. Numpy strings cannot be used in mathematical operations as they are not numeric types.
To resolve this error, you need to convert the numpy string to a numeric type such as a float or an integer before performing the mathematical operation. You can do this using the astype() method provided by numpy.
For example, if you have a numpy string '10' and a float value 5.0, you can convert the string to a float as follows:
```
import numpy as np
# create a numpy string
a = np.str_('10')
# convert the numpy string to a float
b = float(a)
# perform the mathematical operation
c = b - 5.0
print(c)
```
Output:
```
5.0
```
In this example, we convert the numpy string '10' to a float using the float() method and then perform the subtraction operation between the float value and the other float value.
unsupported operand type(s) for -: 'numpy.str_' and 'numpy.str_'
This error occurs when you try to perform a subtraction operation (-) between two numpy string objects. Numpy string objects are not numerical, and therefore cannot be subtracted from each other.
To fix this error, you need to make sure that the objects you are trying to subtract are numerical. You can convert numpy string objects to numerical data types using functions like int() or float().
For example, if you have two numpy string objects '10' and '5', you can convert them to integers and subtract them as follows:
```
a = '10'
b = '5'
result = int(a) - int(b)
print(result)
```
This will output: 5
Alternatively, you can also use the numpy function np.subtract() to perform element-wise subtraction between two numpy arrays.
```
import numpy as np
a = np.array(['10', '20', '30'])
b = np.array(['5', '10', '15'])
result = np.subtract(a.astype(int), b.astype(int))
print(result)
```
This will output: [ 5 10 15]
相关推荐
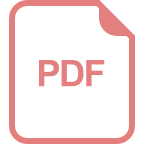
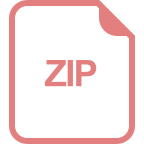









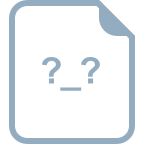
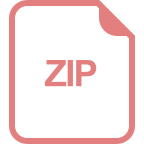