vue 中输入框如何控制只允许输入金额
时间: 2024-05-16 17:14:52 浏览: 17
你可以使用 Vue 的指令来限制用户输入金额。例如,你可以使用 `v-model` 指令绑定输入框的值,然后监听 `input` 事件,在事件处理程序中过滤掉非金额字符。下面是一个例子:
```html
<template>
<div>
<input v-model="amount" @input="filterAmount">
</div>
</template>
<script>
export default {
data() {
return {
amount: ''
}
},
methods: {
filterAmount() {
// 只允许输入数字和小数点
this.amount = this.amount.replace(/[^\d.]/g, '')
// 只保留一个小数点
this.amount = this.amount.replace(/^\./g, '')
this.amount = this.amount.replace(/\.{2,}/g, '.')
// 保留小数点后两位
this.amount = this.amount.replace(/^(\d+)\.(\d{0,2}).*$/, '$1.$2')
}
}
}
</script>
```
这个例子使用正则表达式过滤用户输入的字符,保留金额的格式。你可以根据自己的需求修改这个例子。
相关问题
vue input输入框只可以输入数字
你可以使用Vue的指令v-on:keypress.prevent和v-on:input来实现只能输入数字的效果。
首先,在input标签上添加v-on:keypress.prevent指令来阻止非数字字符的输入:
```html
<input v-on:keypress.prevent="onKeyPress" v-model="inputValue" type="text">
```
然后,在Vue实例中定义onKeyPress方法,判断输入的字符是否为数字:
```javascript
new Vue({
el: '#app',
data: {
inputValue: ''
},
methods: {
onKeyPress: function(event) {
if (!/[0-9]/.test(String.fromCharCode(event.keyCode))) {
event.preventDefault();
}
}
}
})
```
这样就可以实现只能输入数字的效果了。
vue的输入框只能输入数字
,怎么实现?
您可以使用vue中的v-model指令来绑定输入框的数值,并通过设置Vue中的自定义指令来限制输入框只能输入数字。一个简单的实现方法如下:
1. 在Vue中定义一个自定义指令,用于限制输入框只能输入数字:
Vue.directive('number-only', {
bind(el) {
// 只允许输入数字
el.addEventListener('input', (event) => {
event.target.value = event.target.value.replace(/\D/g, '');
})
}
});
2. 在模板中使用v-model指令来绑定输入框的数值,并通过在输入框上使用自定义指令来限制只能输入数字:
<template>
<div>
<input v-model="number" v-number-only />
</div>
</template>
<script>
export default {
data() {
return {
number: ''
}
}
}
</script>
相关推荐
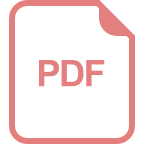
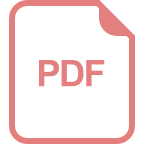












