在jdk11中运用javafx写一个贪吃蛇小游戏
时间: 2024-01-07 15:04:24 浏览: 24
好的,以下是一个简单的贪吃蛇小游戏的 JavaFX 代码示例:
Main.java:
```java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.BorderPane;
import javafx.stage.Stage;
public class Main extends Application {
@Override
public void start(Stage primaryStage) throws Exception{
BorderPane root = new BorderPane();
Scene scene = new Scene(root, 400, 400);
primaryStage.setTitle("贪吃蛇小游戏");
primaryStage.setScene(scene);
primaryStage.show();
Game game = new Game(root);
game.start();
}
public static void main(String[] args) {
launch(args);
}
}
```
Game.java:
```java
import javafx.animation.Animation;
import javafx.animation.KeyFrame;
import javafx.animation.Timeline;
import javafx.scene.layout.BorderPane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
import javafx.util.Duration;
import java.util.Random;
public class Game {
private static final int WIDTH = 20;
private static final int HEIGHT = 20;
private static final int CELL_SIZE = 20;
private static final int SPEED = 200;
private BorderPane root;
private Rectangle[][] cells;
private int[][] map;
private int snakeLength;
private int snakeX;
private int snakeY;
private int directionX;
private int directionY;
private Timeline timeline;
public Game(BorderPane root) {
this.root = root;
cells = new Rectangle[WIDTH][HEIGHT];
map = new int[WIDTH][HEIGHT];
snakeLength = 3;
snakeX = WIDTH / 2;
snakeY = HEIGHT / 2;
directionX = 1;
directionY = 0;
}
public void start() {
init();
timeline = new Timeline(new KeyFrame(Duration.millis(SPEED), event -> {
update();
}));
timeline.setCycleCount(Animation.INDEFINITE);
timeline.play();
}
private void init() {
for (int i = 0; i < WIDTH; i++) {
for (int j = 0; j < HEIGHT; j++) {
Rectangle rect = new Rectangle(i * CELL_SIZE, j * CELL_SIZE, CELL_SIZE, CELL_SIZE);
rect.setFill(Color.WHITE);
rect.setStroke(Color.BLACK);
root.getChildren().add(rect);
cells[i][j] = rect;
}
}
map[snakeX][snakeY] = snakeLength;
updateCell(snakeX, snakeY, Color.GREEN);
createFood();
}
private void update() {
int newSnakeX = snakeX + directionX;
int newSnakeY = snakeY + directionY;
if (newSnakeX < 0 || newSnakeX >= WIDTH || newSnakeY < 0 || newSnakeY >= HEIGHT) {
gameOver();
return;
}
if (map[newSnakeX][newSnakeY] > 0 && map[newSnakeX][newSnakeY] < snakeLength) {
gameOver();
return;
}
if (map[newSnakeX][newSnakeY] == -1) {
snakeLength++;
createFood();
}
updateCell(snakeX, snakeY, Color.WHITE);
updateCell(newSnakeX, newSnakeY, Color.GREEN);
map[snakeX][snakeY] = snakeLength;
snakeX = newSnakeX;
snakeY = newSnakeY;
for (int i = 0; i < WIDTH; i++) {
for (int j = 0; j < HEIGHT; j++) {
if (map[i][j] > 0) {
map[i][j]--;
}
}
}
}
private void gameOver() {
timeline.stop();
}
private void updateCell(int x, int y, Color color) {
cells[x][y].setFill(color);
}
private void createFood() {
Random random = new Random();
int x, y;
do {
x = random.nextInt(WIDTH);
y = random.nextInt(HEIGHT);
} while (map[x][y] != 0);
map[x][y] = -1;
updateCell(x, y, Color.RED);
}
public void setDirection(int directionX, int directionY) {
this.directionX = directionX;
this.directionY = directionY;
}
}
```
在这个示例中,我们使用一个二维矩阵来表示地图,其中 0 表示空格,正整数表示蛇的身体长度,-1 表示食物。游戏通过 Timeline 实现动画效果,每隔一定时间调用 update 方法来更新游戏状态。当蛇撞到墙壁或自身时,游戏结束。可以通过 setDirection 方法来改变蛇的移动方向。
相关推荐
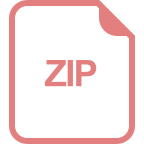
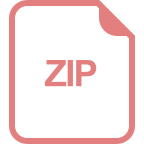
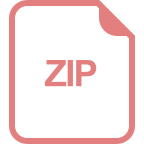














