batchnormalization pytorch
时间: 2023-09-20 18:09:41 浏览: 107
在PyTorch中,Batch Normalization(批标准化)是一种常用的技术,用于加速深度神经网络的训练过程。Batch Normalization的基本概念是对每一个batch中的输入数据进行标准化操作,使其均值为0,方差为1,从而加快网络的收敛速度并提高模型的准确性。
在PyTorch中,可以使用不同维度的Batch Normalization方法来实现批标准化。1d/2d/3d的Batch Normalization适用于不同维度的输入数据。具体来说,1d适用于序列数据,2d适用于图像数据,3d适用于视频数据。PyTorch提供了相应的函数和类来实现这些方法。
在使用Batch Normalization时,需要注意输入数据的维度和计算方式。根据不同的维度,PyTorch提供了相应的输入和计算方式。同时,还可以参考PyTorch的官方文档和代码示例来实现批标准化。
总结来说,在PyTorch中,Batch Normalization是一种用于加速深度神经网络训练的技术,通过对每一个batch中的输入数据进行标准化操作,提高模型的准确性。PyTorch提供了不同维度的Batch Normalization方法来适应不同类型的数据,并提供了相应的函数和类来实现这些方法。可以参考官方文档和代码示例来了解更多细节。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
相关问题
Batch Normalization代码pytorch
Sure! Here's an example of how to implement Batch Normalization in PyTorch:
```python
import torch
import torch.nn as nn
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.fc1 = nn.Linear(10, 20)
self.bn1 = nn.BatchNorm1d(20)
self.fc2 = nn.Linear(20, 2)
def forward(self, x):
x = self.fc1(x)
x = self.bn1(x)
x = torch.relu(x)
x = self.fc2(x)
return x
# Create an instance of the network
net = Net()
# Define your input data
input_data = torch.randn(5, 10)
# Pass the input data through the network
output = net(input_data)
# Print the output
print(output)
```
In this example, we define a simple neural network with one hidden layer and one output layer. The Batch Normalization layer is applied after the first fully connected layer (`self.fc1`) and before the activation function (`torch.relu`). The `nn.BatchNorm1d` class is used to create the Batch Normalization layer.
Please note that this is just a basic example to demonstrate how to use Batch Normalization in PyTorch. In real-world scenarios, you may need to adjust the network architecture and hyperparameters according to your specific task.
batch normalization的pytorch源码
下面是 Batch Normalization 在 PyTorch 中的源代码:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class BatchNorm(nn.Module):
def __init__(self, num_features, eps=1e-5, momentum=0.1):
super(BatchNorm, self).__init__()
self.num_features = num_features
self.eps = eps
self.momentum = momentum
self.weight = nn.Parameter(torch.ones(num_features))
self.bias = nn.Parameter(torch.zeros(num_features))
self.register_buffer('running_mean', torch.zeros(num_features))
self.register_buffer('running_var', torch.ones(num_features))
def forward(self, x):
if self.training:
mean = x.mean(dim=0)
var = x.var(dim=0, unbiased=False)
self.running_mean = (1 - self.momentum) * self.running_mean + self.momentum * mean
self.running_var = (1 - self.momentum) * self.running_var + self.momentum * var
x = (x - mean) / (torch.sqrt(var + self.eps))
else:
x = (x - self.running_mean) / (torch.sqrt(self.running_var + self.eps))
return x * self.weight + self.bias
```
这里定义了一个名为 `BatchNorm` 的类,它继承自 `nn.Module`。在 `__init__` 方法中,我们初始化了权重 `weight` 和偏置 `bias` 参数,以及用于记录均值和方差的缓冲区 `running_mean` 和 `running_var`。`eps` 是一个很小的常数,用于稳定计算的分母。
在 `forward` 方法中,我们首先计算输入 `x` 的均值和方差,并更新 `running_mean` 和 `running_var`。然后,根据当前模式(训练或推断),我们使用均值和方差对输入进行归一化。最后,我们将归一化后的结果乘以权重 `weight` 并加上偏置 `bias`,得到最终的输出。
这段代码提供了一个基本的 Batch Normalization 的实现,但并未涵盖所有细节和特殊情况。在实际使用中,你可能需要考虑更多因素,如 `track_running_stats` 参数、不同维度的归一化等。如果需要更完整和高效的 Batch Normalization 实现,建议使用 PyTorch 内置的 `nn.BatchNorm2d` 或 `nn.BatchNorm1d` 层。
阅读全文
相关推荐
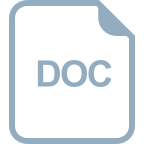
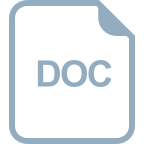
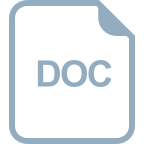
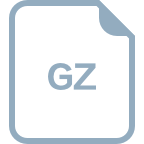



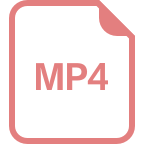
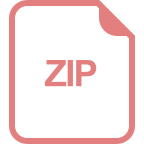







