std::unordered_map < std::string, SendToWeb> _sendWebInfoToEle 遍历删除
时间: 2024-01-19 13:03:47 浏览: 33
如果你想遍历`std::unordered_map`中的所有元素并删除它们,可以使用以下代码:
```cpp
for (auto it = _sendWebInfoToEle.begin(); it != _sendWebInfoToEle.end(); ) {
it = _sendWebInfoToEle.erase(it);
}
```
这里使用了一个迭代器`it`来遍历`_sendWebInfoToEle`中的所有元素,如果你直接在循环中使用`erase()`方法来删除元素,会导致迭代器失效,因此需要使用特殊的技巧:先将迭代器移动到下一个位置,再使用`erase()`方法来删除当前位置的元素。
注意,这里的`it`在循环中不需要自增,因为在`erase()`方法中已经将它设置为了下一个位置。另外,如果你想在遍历中对元素进行操作,可以在循环中使用`it->first`和`it->second`来访问键和值。
相关问题
std::unordered_map<std::string,std::string>
std::unordered_map<std::string, std::string>是C++标准库中的一个容器,用于存储键值对。是一个哈希表实现的关联容器,可以根据键快速查找对应的值。其中,键和值都是字符串类型。
unordered_map使用哈希函数将键映射到桶中,以实现快速的查找和插入操作。它的插入、删除和查找操作的平均时间复杂度都是常数级别的,即O(1)。
unordered_map中的键是唯一的,每个键只能对应一个值。如果插入了重复的键,则新值会覆盖旧值。
unordered_map提供了丰富的成员函数和操作符重载,可以方便地进行元素的插入、删除、查找和遍历等操作。
遍历std::unordered_map
遍历std::unordered_map可以使用迭代器来实现。以下是一种常见的遍历方式:
```cpp
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<int, std::string> myMap = {{1, "apple"}, {2, "banana"}, {3, "orange"}};
// 使用迭代器遍历unordered_map
for (auto it = myMap.begin(); it != myMap.end(); ++it) {
std::cout << "Key: " << it->first << ", Value: " << it->second << std::endl;
}
return 0;
}
```
在上述代码中,我们首先创建了一个std::unordered_map对象myMap,并初始化了一些键值对。然后,我们使用for循环和迭代器来遍历myMap。迭代器it指向当前键值对,通过it->first和it->second可以获取键和值。
相关推荐
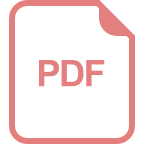
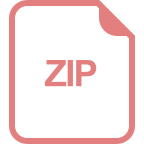












