class Player { constructor(x, y, radius, color, vector) { this.x = x; this.y = y; this.radius = radius; this.color = color; this.vector = vector; this.life = 3;//玩家生命值 } draw(ctx) { ctx.save(); ctx.beginPath(); ctx.fillStyle = this.color; ctx.arc(this.x, this.y, this.radius, 0, Math.PI * 2, false); ctx.fill(); ctx.closePath(); ctx.restore(); } resize(x, y) { this.x = x; this.y = y; } } class Enemy { constructor(x, y, radius, color, vector) { this.x = x; this.y = y; this.radius = radius; this.color = color; this.vector = vector; } draw(ctx) { ctx.save(); ctx.beginPath(); ctx.fillStyle = this.color; ctx.arc(this.x, this.y, this.radius, 0, Math.PI * 2, false); ctx.fill(); ctx.closePath(); ctx.restore(); } update() { this.x += this.vector.x; this.y += this.vector.y; } } 要为玩家添加生命值。判断敌人与玩家是否撞击,是则玩家的生命值减1当玩家生命值减到0时,游戏结束。
时间: 2024-03-03 18:51:41 浏览: 35
可以在 `Player` 类中添加一个 `life` 属性来表示玩家的生命值,并将其初始化为 3。然后在 `Enemy` 类中添加一个 `checkCollision()` 方法来检测敌人与玩家是否撞击,如果撞击,则将玩家的生命值减 1。在 `Player` 类中添加一个 `checkGameOver()` 方法来检测玩家生命值是否减为 0,如果减为 0,则游戏结束。
下面是修改后的代码:
```
class Player {
constructor(x, y, radius, color, vector) {
this.x = x;
this.y = y;
this.radius = radius;
this.color = color;
this.vector = vector;
this.life = 3; // 玩家生命值
}
draw(ctx) {
ctx.save();
ctx.beginPath();
ctx.fillStyle = this.color;
ctx.arc(this.x, this.y, this.radius, 0, Math.PI * 2, false);
ctx.fill();
ctx.closePath();
ctx.restore();
}
resize(x, y) {
this.x = x;
this.y = y;
}
checkGameOver() {
if (this.life === 0) {
// 游戏结束
console.log('Game over');
}
}
}
class Enemy {
constructor(x, y, radius, color, vector) {
this.x = x;
this.y = y;
this.radius = radius;
this.color = color;
this.vector = vector;
}
draw(ctx) {
ctx.save();
ctx.beginPath();
ctx.fillStyle = this.color;
ctx.arc(this.x, this.y, this.radius, 0, Math.PI * 2, false);
ctx.fill();
ctx.closePath();
ctx.restore();
}
update() {
this.x += this.vector.x;
this.y += this.vector.y;
}
checkCollision(player) {
const distance = Math.sqrt((player.x - this.x) ** 2 + (player.y - this.y) ** 2);
if (distance < player.radius + this.radius) {
// 玩家与敌人撞击,玩家生命值减1
player.life--;
console.log('Life:', player.life);
}
}
}
```
相关推荐
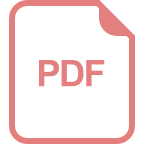
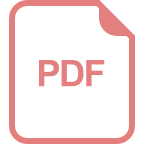
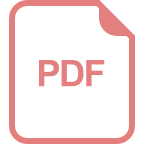















