PDFSharp在PDF中插入图片
时间: 2023-12-08 16:04:19 浏览: 177
要在PDF中插入图片,可以使用PDFSharp库中的XImage类。以下是一个示例代码:
```csharp
// 创建一个PDF文档
PdfDocument document = new PdfDocument();
// 添加一页
PdfPage page = document.AddPage();
// 加载图片
XImage image = XImage.FromFile("image.jpg");
// 获取页面的绘图对象
XGraphics gfx = XGraphics.FromPdfPage(page);
// 将图片绘制到页面上
gfx.DrawImage(image, 100, 100);
// 保存PDF文档
document.Save("output.pdf");
```
在这个示例中,我们首先创建一个PDF文档和一页。然后,使用XImage类加载要插入的图像。接下来,我们获取页面的绘图对象,并使用DrawImage方法将图像绘制到页面上。最后,我们保存PDF文档。
这只是一个简单的示例,你可以根据需要调整图像的位置和大小。同时,如果你需要更多的控制,可以使用PDFSharp提供的其他类和方法来实现更复杂的操作。
相关问题
使用C# PDFsharp库将图片插入到PDF中的完整示例?
在C#中,使用PDFsharp库将图片插入到PDF文件中,可以按照以下步骤编写一个简单的示例:
```csharp
using System;
using System.IO;
using PdfSharp.Drawing;
using PdfSharp.Pdf;
namespace InsertImageIntoPdf
{
class Program
{
static void Main(string[] args)
{
// 读取图片
Bitmap image = new Bitmap("path_to_your_image.jpg"); // 替换为你的图片路径
// 创建一个新的PDF文档
Document document = new Document();
PdfPage page = document.AddPage();
// 设置页面大小和布局
XUnit pageSizeWidth = 595.28; // 美国标准A4纸尺寸
XUnit pageSizeHeight = 841.89;
page.Margins = new Margins(0, 0, pageSizeWidth, pageSizeHeight);
// 将图片转换为XGraphics对象
XGraphics gfx = XGraphics.FromPdfPage(page);
ImageXObject imgXObject = gfx.CreateImageObject(image);
// 定义插入图像的位置
XPoint position = new XPoint(pageSizeWidth / 2 - imgXObject.Width / 2, pageSizeHeight / 2 - imgXObject.Height / 2);
// 插入图片
gfx.DrawImage(imgXObject, position);
// 保存PDF文件
string outputFilePath = "output.pdf";
using (FileStream stream = File.Create(outputFilePath))
document.Save(stream);
Console.WriteLine($"Image inserted successfully into PDF at {outputFilePath}.");
Console.ReadKey();
}
}
}
```
在这个例子中,你需要替换`"path_to_your_image.jpg"`为你实际的图片路径。这个程序会创建一个新的PDF文档,添加一页,并将指定的图片放置在页面中心位置。
使用C# PDFsharp库将图片插入并填充到PDF占位符中的完整示例?
在C#中,使用PDFsharp库将图片插入PDF文档并填充到特定位置通常涉及以下几个步骤:
```csharp
using iTextSharp.text;
using iTextSharp.text.pdf;
using System.IO;
// 创建PDF文档实例
Document document = new Document();
PdfWriter.GetInstance(document, new FileStream("output.pdf", FileMode.Create));
// 打开PDF
document.Open();
// 定义图像路径和占位符的位置
string imagePath = "image.jpg";
int xPosition = 100; // 横向偏移
int yPosition = 500; // 纵向偏移
// 加载图片
Image image = Image.GetInstance(imagePath);
// 设置图片缩放比例,如果需要的话
float scale = 1f; // 如果你想保持原始尺寸,可以设置为1
if (image.Width > 100 || image.Height > 100) {
scale = 100 / Math.Max(image.Width, image.Height);
image.ScaleAbsolute(scale, scale);
}
// 获取占位符的Box对象
Rectangle position = new Rectangle(xPosition, yPosition, 200, 150); // 占位符大小和位置
// 将图片添加到页面上,并填充到指定位置
ColumnText.ShowTextAligned(document.GetDirectContent(), Element.ALIGN_CENTER, image, position.Left, position.Top, 0);
// 关闭PDF
document.Close();
阅读全文
相关推荐




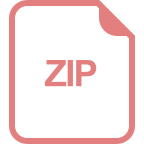
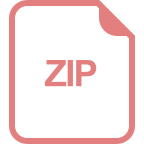
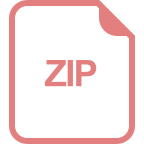
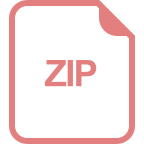
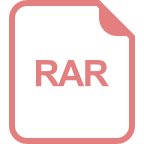



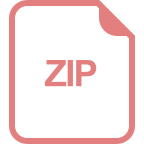
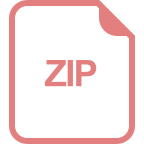
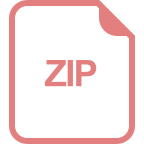