export default { name: "AppNavbar", data() { return { activeIndex: "1", activeIndex2: "1", }; }, methods: { logout(){ localStorage.removeItem("token") localStorage.removeItem("id") this.$router.push("/") }, async deleteUser(){ const userid = localStorage.getItem('id') this.$confirm('确定要注销吗?','提示',{ confirmButtonText: "确定", cancelButtonText: "取消", type: "warning", }).then(async () => { try { // 根据需要替换为你的 API 地址 const apiUrl = `http://127.0.0.1:5001/users/${userid}`; const token = localStorage.getItem("token"); // 从 localStorage 中获取存储的令牌 const response = await axios.delete(apiUrl, { headers: { Authorization: `Bearer ${token}`, }, }); if (response.status === 200) { this.$message({ 'message':"注销成功!", 'type':'success' }) console.log(response.data.message); // 在控制台输出成功删除的消息 this.$router.push('/') // 在这里添加其他操作,例如更新界面或通知用户 } } catch (error) { console.error("Error deleting user:", error); // 在这里处理错误,例如通知用户删除失败 } }) .catch(() => { this.$message.info("取消注销"); this.$router.push('/main/userinfo') }); }, handleSelect(key, keyPath) { console.log(key, keyPath); }, }, };什么意思
时间: 2023-12-06 09:43:22 浏览: 190
这是一个 Vue.js 组件,名为 "AppNavbar",包含了一个 data() 方法和多个 methods() 方法。其中,data() 方法返回了两个变量 activeIndex 和 activeIndex2,methods() 方法包括了以下几个:
- logout(): 当用户点击注销按钮时,从本地存储中移除 token 和 id,并将页面跳转至首页。
- deleteUser(): 当用户点击注销账户按钮时,弹出确认框,确认后向服务器发送删除用户请求,如果删除成功则提示用户注销成功,并且页面跳转至首页。
- handleSelect(): 当用户在导航栏上选择了某个选项时,会在控制台输出选项对应的 key 和 keyPath。
这些方法用于实现一个基本的导航栏功能,可以在 Vue.js 应用中使用。
相关问题
import { mapState } from "vuex"; export default { name: 'flowerList', data() { return { flowerPage: [], page: { currentPage: 1, pageSize: 8, total: 0, }, activeIndex: 0, } },
这是Vue.js中定义一个名为"flowerList"的组件,并引入了vuex中的mapState方法。data中定义了组件的数据属性:
- flowerPage:表示当前页的数据数组
- page:表示分页相关的对象,包括当前页码currentPage、每页显示数量pageSize和总记录数total
- activeIndex:表示当前选中的页码
通过return语句返回这些数据属性,使得这些属性可以在组件中被访问和使用。
怎么把<script> export default { data() { return { activeIndex: '1', activeIndex2: '1' }; }, methods: { handleSelect(key, keyPath) { console.log(key, keyPath); } } } </script>这串代码改成<script setup格式的>
好的,下面是将原来的 `<script>` 标签改写成 `<script setup>` 标签的代码:
```html
<template>
<div>
<el-menu :default-active="activeIndex" mode="horizontal" @select="handleSelect">
<el-menu-item index="1">导航一</el-menu-item>
<el-menu-item index="2">导航二</el-menu-item>
<el-menu-item index="3">导航三</el-menu-item>
</el-menu>
<el-menu :default-active="activeIndex2" mode="vertical" @select="handleSelect">
<el-menu-item index="1">导航一</el-menu-item>
<el-menu-item index="2">导航二</el-menu-item>
<el-menu-item index="3">导航三</el-menu-item>
</el-menu>
</div>
</template>
<script setup>
import { getCurrentInstance } from 'vue';
const { proxy } = getCurrentInstance();
const activeIndex = '1';
const activeIndex2 = '1';
const handleSelect = (key, keyPath) => {
console.log(key, keyPath);
}
// 将数据和方法暴露给模板使用
return {
activeIndex,
activeIndex2,
handleSelect
}
</script>
```
在上面的代码中,我们使用了 `getCurrentInstance()` 函数来获取组件实例,然后使用 `proxy` 属性获取组件实例的代理。这个代理对象可以让我们像在 `data()` 和 `methods()` 中一样定义和访问数据和方法。
需要注意的是,在 `<script setup>` 中,我们不能像在 `<script>` 中那样使用 `export default` 导出选项对象,而是需要使用 `return` 语句来返回一个对象,该对象包含了需要暴露给模板的数据和方法。
阅读全文
相关推荐
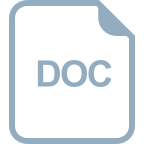
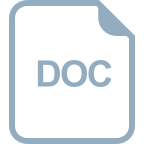
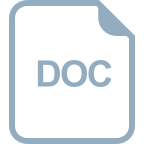









const activeIndex=Vue.ref(0);
// console.log(activeIndex.valuea);
function toggleActive(index){
if(activeIndex.value===index){
activeIndex.value=null;
}else{
activeIndex.value=index;
console.log(activeIndex);
console.log(activeIndex.value);
}
const lis =document.querySelectorAll(".clearfix li")
for(let i=0;i{{option.category_title}}
和
{{option2.subtitle}}
{{option2.title}}
{{option2.summary}}
{{option2.subtitle}}
{{option2.title}}
{{option2.summary}}
实现tab切换




