Java开发微信小程序支付(支付,提现,退款)代码
时间: 2023-10-15 11:02:12 浏览: 157
以下是使用 Java 开发微信小程序支付、提现、退款的示例代码:
1. 发起支付请求:
```java
// 构建支付请求参数
Map<String, String> params = new HashMap<>();
params.put("appid", "小程序的appid");
params.put("mch_id", "商户号");
params.put("nonce_str", "随机字符串");
params.put("body", "商品描述");
params.put("out_trade_no", "商户订单号");
params.put("total_fee", "订单总金额,单位为分");
params.put("spbill_create_ip", "调用微信支付API的机器IP");
params.put("notify_url", "接收微信支付异步通知回调的地址");
params.put("trade_type", "支付类型,如JSAPI");
params.put("openid", "用户的openid");
// 生成签名
String sign = WXPayUtil.generateSignature(params, "支付密钥");
// 添加签名到请求参数中
params.put("sign", sign);
// 发起支付请求
WXPay wxpay = new WXPay(new WXPayConfig(), true, true);
Map<String, String> result = wxpay.unifiedOrder(params);
```
2. 接收支付结果:
```java
// 接收支付结果通知
String xmlData = "<xml>...</xml>";
Map<String, String> notifyMap = WXPayUtil.xmlToMap(xmlData);
// 验证支付结果
boolean isSignatureValid = WXPayUtil.isSignatureValid(notifyMap, "支付密钥");
if (isSignatureValid) {
String returnCode = notifyMap.get("return_code");
String resultCode = notifyMap.get("result_code");
if ("SUCCESS".equals(returnCode) && "SUCCESS".equals(resultCode)) {
// 支付成功
}
}
```
3. 发起提现请求:
```java
// 构建提现请求参数
Map<String, String> params = new HashMap<>();
params.put("mch_appid", "小程序的appid");
params.put("mchid", "商户号");
params.put("nonce_str", "随机字符串");
params.put("partner_trade_no", "商户订单号");
params.put("openid", "用户的openid");
params.put("check_name", "NO_CHECK");
params.put("amount", "提现金额,单位为分");
params.put("desc", "提现描述");
params.put("spbill_create_ip", "调用接口的机器IP");
// 生成签名
String sign = WXPayUtil.generateSignature(params, "支付密钥");
// 添加签名到请求参数中
params.put("sign", sign);
// 发起提现请求
WXPay wxpay = new WXPay(new WXPayConfig(), true, true);
Map<String, String> result = wxpay.transfer(params);
```
4. 发起退款请求:
```java
// 构建退款请求参数
Map<String, String> params = new HashMap<>();
params.put("appid", "小程序的appid");
params.put("mch_id", "商户号");
params.put("nonce_str", "随机字符串");
params.put("out_trade_no", "商户订单号");
params.put("out_refund_no", "商户退款单号");
params.put("total_fee", "订单总金额,单位为分");
params.put("refund_fee", "退款金额,单位为分");
// 生成签名
String sign = WXPayUtil.generateSignature(params, "支付密钥");
// 添加签名到请求参数中
params.put("sign", sign);
// 发起退款请求
WXPay wxpay = new WXPay(new WXPayConfig(), true, true);
Map<String, String> result = wxpay.refund(params);
```
需要注意的是,以上代码中的 `WXPayConfig` 类需要自行实现,用于配置微信支付的 API 地址、证书等信息。另外,示例代码中的参数都需要根据实际情况进行替换。
阅读全文
相关推荐


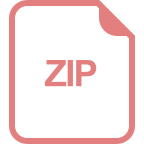
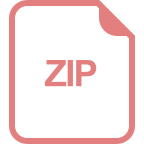
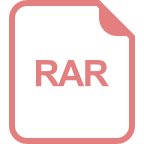
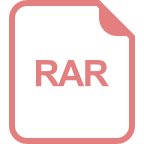
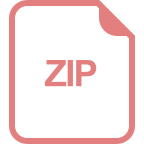
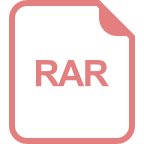
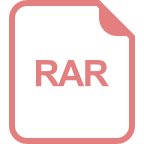
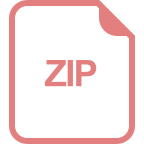
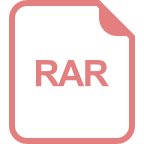
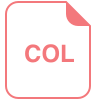
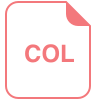
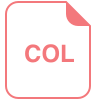