二、试设计一个“111”序列检测器。要求当连续输入三个或三个以上“1”时,输出为“1”,否则输出为“0”。要求先画出状态图,再写出VHDL代码,用mealy型状态机实现。
时间: 2024-03-31 14:38:20 浏览: 244
首先画出状态图,如下所示:

在状态图中,S0、S1、S2 分别表示输入的 1 的个数为 0、1、2 的状态,S3 表示输入的 1 的个数已经达到 3 个,输出为 1,即检测到了 111 序列,而 S4 表示输入的 1 的个数已经大于等于 3 个,但并不是连续的 111 序列,即输出为 0。
根据状态图,我们可以写出 VHDL 代码,如下所示:
```vhdl
library ieee;
use ieee.std_logic_1164.all;
entity sequence_detector is
port(
clk: in std_logic;
reset: in std_logic;
input: in std_logic;
output: out std_logic
);
end entity sequence_detector;
architecture mealy of sequence_detector is
-- 状态定义
type state_type is (S0, S1, S2, S3, S4);
signal state: state_type;
begin
-- 状态转移逻辑
process(clk, reset)
begin
if reset = '1' then -- 复位
state <= S0;
elsif rising_edge(clk) then -- 上升沿时进行状态转移
case state is
when S0 => -- 输入为 0
if input = '1' then
state <= S1;
else
state <= S0;
end if;
when S1 => -- 输入为 1
if input = '1' then
state <= S2;
else
state <= S0;
end if;
when S2 => -- 输入为 1
if input = '1' then
state <= S3;
else
state <= S0;
end if;
when S3 => -- 检测到 111 序列
if input = '1' then
state <= S4;
else
state <= S0;
end if;
when S4 => -- 输入为 1 或 0 都不影响输出
state <= S4;
end case;
end if;
end process;
-- 输出逻辑
output <= '1' when state = S3 else '0';
end architecture mealy;
```
在代码中,我们使用 Mealy 型状态机实现了 111 序列检测器。其中,输入信号为 `input`,输出信号为 `output`。时钟信号为 `clk`,复位信号为 `reset`。在状态转移逻辑中,我们根据当前状态和输入信号的值,进行状态转移。在输出逻辑中,当检测到 111 序列时,输出为 1,否则为 0。
阅读全文
相关推荐
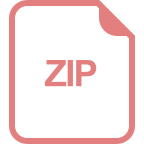
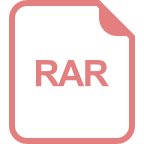
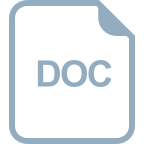
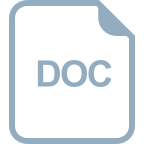
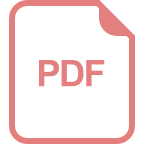
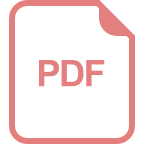
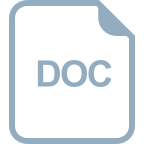
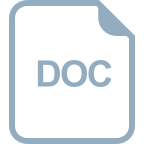
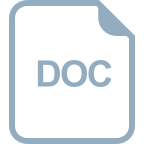
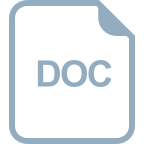
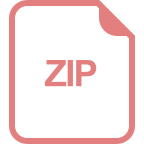
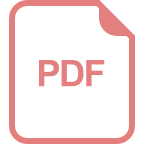
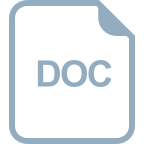
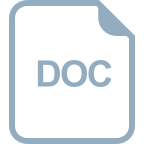
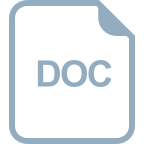
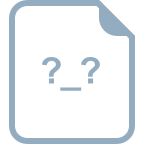
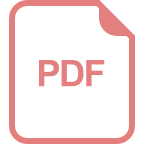