用 C语言实现一个简单的 shell
时间: 2023-05-29 10:04:23 浏览: 64
以下是一个简单的 shell 的 C 语言代码实现,它可以执行 shell 命令和内置命令:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/wait.h>
#define MAX_COMMAND_LENGTH 100
#define MAX_NUM_ARGUMENTS 10
char *builtin_commands[] = {"cd", "exit"};
void cd_command(char *directory) {
if (directory == NULL) {
fprintf(stderr, "cd: expected argument to \"cd\"\n");
} else {
if (chdir(directory) != 0) {
perror("cd");
}
}
}
void execute_command(char **args) {
pid_t pid = fork();
if (pid == 0) { // child process
if (execvp(args[0], args) == -1) {
perror("execute_command");
}
exit(EXIT_FAILURE);
} else if (pid < 0) {
perror("execute_command");
} else { // parent process
int status;
do {
waitpid(pid, &status, WUNTRACED);
} while (!WIFEXITED(status) && !WIFSIGNALED(status));
}
}
void execute_builtin_command(char **args) {
if (strcmp(args[0], "cd") == 0) {
cd_command(args[1]);
} else if (strcmp(args[0], "exit") == 0) {
exit(EXIT_SUCCESS);
}
}
int main() {
char command[MAX_COMMAND_LENGTH];
char *args[MAX_NUM_ARGUMENTS];
while (1) {
printf("> ");
if (fgets(command, MAX_COMMAND_LENGTH, stdin) == NULL) {
break;
}
int num_args = 0;
args[num_args] = strtok(command, " \t\n");
while (args[num_args] != NULL && num_args < MAX_NUM_ARGUMENTS - 1) {
num_args++;
args[num_args] = strtok(NULL, " \t\n");
}
args[num_args] = NULL;
if (args[0] == NULL) {
continue;
}
if (strcmp(args[0], "!!") == 0) {
printf("error: no previous command to execute\n");
continue;
}
if (strcmp(args[0], "cd") == 0 || strcmp(args[0], "exit") == 0) {
execute_builtin_command(args);
} else {
execute_command(args);
}
}
return 0;
}
```
该 shell 可以执行如下命令:
- 内置命令 `cd`,用于改变当前工作目录。例如:`cd /home/user/documents`
- 内置命令 `exit`,用于退出 shell。例如:`exit`
- 外部命令,例如:`ls -l`,`gcc -o hello hello.c` 等。
除此之外,该 shell 还支持如下功能:
- `!!` 命令,用于执行上一条命令。例如:`ls -l`,`!!`
- 忽略空白命令行。例如,直接按回车键会被忽略。
- 支持使用空格和制表符分隔命令行参数。
注意,该 shell 的实现仅供参考,可能存在漏洞和不足之处。
相关推荐
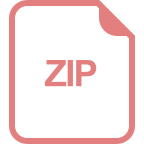
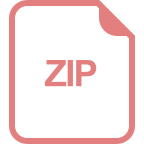














