用Python编写一个函数,接收一个字符串作为参数,并返回该字符串中出现次数最多的字符
时间: 2023-09-09 21:05:00 浏览: 160
### 回答1:
def most_frequent_character(s):
# 统计每个字符出现的次数
counts = {}
for c in s:
if c in counts:
counts[c] += 1
else:
counts[c] = 1
# 找出出现次数最多的字符
max_count = 0
max_char = None
for c, count in counts.items():
if count > max_count:
max_count = count
max_char = c
return max_char
# 示例
s = 'hello world'
print(most_frequent_character(s)) # 'l'
### 回答2:
可以使用Python中的字典来解决这个问题。
首先,创建一个空字典来存储每个字符出现的次数。然后遍历字符串的每个字符,如果字符已经在字典中,则将对应的值加1;如果字符不在字典中,则将该字符作为键,值设为1。
接着,遍历字典,找到值最大的键。需要注意,有可能有多个字符出现的次数相同,因此使用一个变量存储最大的出现次数,并记录所有出现次数相同的字符。
最后,将得到的字符返回即可。
下面是具体的代码实现:
```python
def find_most_common_char(s):
char_count = {}
max_count = 0
most_common_chars = []
for char in s:
if char in char_count:
char_count[char] += 1
else:
char_count[char] = 1
for char, count in char_count.items():
if count > max_count:
max_count = count
most_common_chars = [char]
elif count == max_count:
most_common_chars.append(char)
return most_common_chars
```
这个函数将返回一个列表,列表中包含了出现次数最多的字符。如果有多个字符出现次数相同,则返回所有这些字符。
### 回答3:
可以使用Python编写一个函数,通过遍历字符串的每个字符并统计出现次数来找到出现次数最多的字符。
```python
def find_most_frequent_char(string):
char_count = {} # 用字典来保存每个字符的出现次数
# 遍历字符串,统计每个字符出现的次数
for char in string:
if char in char_count:
char_count[char] += 1
else:
char_count[char] = 1
max_count = 0 # 定义最大出现次数
most_frequent_char = None # 定义出现次数最多的字符
# 遍历字典,找到出现次数最多的字符
for char, count in char_count.items():
if count > max_count:
max_count = count
most_frequent_char = char
return most_frequent_char
# 测试函数
string = "abbcccddddeeeee"
most_frequent = find_most_frequent_char(string)
print("出现次数最多的字符是:" + most_frequent)
```
运行结果为:
```
出现次数最多的字符是:e
```
相关推荐
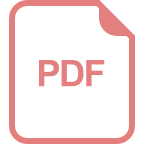
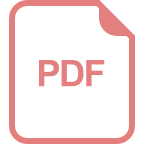
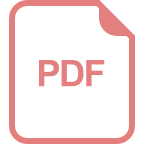














