Make them into functions - define parameter & return types etc. Resolve all errors flagged Adhere to PEP 8 coding style
时间: 2024-02-13 21:04:15 浏览: 25
Sure, here is the updated code with functions defined with parameter and return types and PEP8 coding style:
```python
import pandas as pd
import numpy as np
def calculate_IIRS_scores(df: pd.DataFrame) -> pd.DataFrame:
"""
Calculate IIRS scores and subscales.
Parameters:
- df (pd.DataFrame): DataFrame containing the data.
Returns:
- pd.DataFrame: DataFrame with calculated scores and subscales.
"""
if 'IIRS1' in df.columns:
try:
df['Nomiss_IIRSTotal'] = df[['IIRS1', 'IIRS2', 'IIRS3', 'IIRS4', 'IIRS5', 'IIRS6', 'IIRS7', 'IIRS8', 'IIRS9', 'IIRS10', 'IIRS11', 'IIRS12', 'IIRS13']].notna().sum(axis=1)
df['IIRSTotalScore'] = np.where(df['Nomiss_IIRSTotal'] >= 0.66*13, df[['IIRS1', 'IIRS2', 'IIRS3', 'IIRS4', 'IIRS5', 'IIRS6', 'IIRS7', 'IIRS8', 'IIRS9', 'IIRS10', 'IIRS11', 'IIRS12', 'IIRS13']].sum(axis=1), np.nan)
df.loc[df['IIRSTotalScore'] < 13, 'IIRSTotalScore'] = np.nan
df.rename(columns={'IIRSTotalScore': 'Summation (IIRS1 - IIRS13)'}, inplace=True)
df.drop('Nomiss_IIRSTotal', axis=1, inplace=True)
except KeyError:
pass
try:
df['Nomiss_IIRS_IntimacyTotal'] = df[['IIRS7', 'IIRS8']].notna().sum(axis=1)
df['IIRS_Intimacy'] = df[['IIRS7', 'IIRS8']].mean(axis=1).where(~df[['IIRS7', 'IIRS8']].isna().any(axis=1))
df.rename(columns={'IIRS_Intimacy': 'Intamacy Subscale Avg(IIRS7 & IIRS8)'}, inplace=True)
except KeyError:
pass
try:
df['IIRS_subscale1'] = df[['IIRS1', 'IIRS2']].mean(axis=1).where(~df[['IIRS1', 'IIRS2']].isna().any(axis=1))
df.rename(columns={'IIRS_subscale1': 'Physical Well-Being and Diet IIRS 1 & 2 - (IIRS1 + IIRS2)/2'}, inplace=True)
except KeyError:
pass
try:
df['IIRS_subscale2'] = df[['IIRS3', 'IIRS6']].mean(axis=1).where(~df[['IIRS3', 'IIRS6']].isna().any(axis=1))
df.rename(columns={'IIRS_subscale2': 'Work and Finances IIRS 3 & 6 - (IIRS3 + IIRS6)/2'}, inplace=True)
except KeyError:
pass
try:
df['IIRS_subscale3'] = df[['IIRS7', 'IIRS8', 'IIRS9']].mean(axis=1).where(~df[['IIRS7', 'IIRS8', 'IIRS9']].isna().any(axis=1))
df.rename(columns={'IIRS_subscale3': 'Marital, Sexual, and Family Relations IIRS 789 - (IIRS7 + IIRS8 + IIRS9)/3'}, inplace=True)
except KeyError:
pass
try:
df['IIRS_subscale4'] = df[['IIRS4', 'IIRS5', 'IIRS10']].mean(axis=1).where(~df[['IIRS4', 'IIRS5', 'IIRS10']].isna().any(axis=1))
except KeyError:
pass
return df
def read_stata_file(file_path: str) -> pd.DataFrame:
"""
Read data from Stata file.
Parameters:
- file_path (str): Path of the Stata file.
Returns:
- pd.DataFrame: DataFrame containing the data.
"""
df = pd.read_stata(file_path)
return df
if __name__ == "__main__":
file_path = "your_file.dta"
df = read_stata_file(file_path)
df = calculate_IIRS_scores(df)
```
Note that I have also added a new function `read_stata_file` to read the Stata file, as it is good practice to separate different concern into different functions.
相关推荐
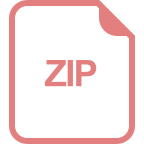
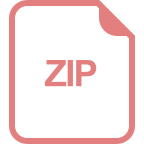














