用c语言写一个500x500的坐标系随机取点要求两个点间距至少为5且离边界距离至少2.5
时间: 2024-01-24 17:17:19 浏览: 23
以下是一个简单的C语言程序,用于生成一个500x500的坐标系,并在其中随机选择一些点,使得每对点之间的距离至少为5,且每个点距离边界至少有2.5的距离。
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define WIDTH 500
#define HEIGHT 500
#define MIN_DIST 5
#define MIN_BORDER_DIST 2.5
struct Point {
int x;
int y;
};
double getDistance(struct Point p1, struct Point p2) {
int dx = p1.x - p2.x;
int dy = p1.y - p2.y;
return sqrt(dx * dx + dy * dy);
}
int isValidPoint(struct Point p, struct Point points[], int n) {
if (p.x < MIN_BORDER_DIST || p.x > WIDTH - MIN_BORDER_DIST ||
p.y < MIN_BORDER_DIST || p.y > HEIGHT - MIN_BORDER_DIST) {
return 0;
}
for (int i = 0; i < n; i++) {
if (getDistance(p, points[i]) < MIN_DIST) {
return 0;
}
}
return 1;
}
int main() {
srand(time(NULL));
int n = 100; // 生成100个点
struct Point points[n];
int count = 0;
while (count < n) {
struct Point p = {rand() % WIDTH + 1, rand() % HEIGHT + 1};
if (isValidPoint(p, points, count)) {
points[count++] = p;
}
}
// 输出点的坐标
for (int i = 0; i < n; i++) {
printf("%d %d\n", points[i].x, points[i].y);
}
return 0;
}
```
该程序使用了结构体来表示一个点,使用了getDistance函数来计算两个点之间的距离,使用了isValidPoint函数来检查一个点是否合法。程序通过随机生成点,并检查它们是否符合要求来生成给定数量的点。
相关推荐
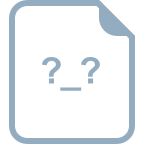














