c语言动态顺序表输入输出元素逆置
时间: 2023-05-30 10:05:24 浏览: 119
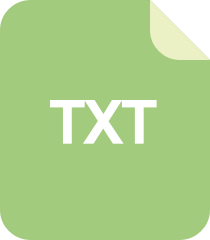
用C语言实现顺序表的逆置

动态顺序表是指可以动态增加或删除元素的顺序表,通常使用动态分配内存的方式实现。在C语言中,动态顺序表可以使用结构体和指针实现。
输入元素:
```c
#include <stdio.h>
#include <stdlib.h>
#define LIST_INIT_SIZE 10
#define LIST_INCREMENT 5
typedef struct {
int *elem;
int length;
int listsize;
} SqList;
int InitList(SqList *L) {
L->elem = (int *) malloc(LIST_INIT_SIZE * sizeof(int));
if (!L->elem) {
return 0;
}
L->length = 0;
L->listsize = LIST_INIT_SIZE;
return 1;
}
int ListInsert(SqList *L, int i, int e) {
if (i < 1 || i > L->length + 1) {
return 0;
}
if (L->length >= L->listsize) {
int *newbase = (int *) realloc(L->elem, (L->listsize + LIST_INCREMENT) * sizeof(int));
if (!newbase) {
return 0;
}
L->elem = newbase;
L->listsize += LIST_INCREMENT;
}
int *p = &L->elem[i - 1];
for (int *q = &L->elem[L->length - 1]; q >= p; --q) {
*(q + 1) = *q;
}
*p = e;
++L->length;
return 1;
}
void InputList(SqList *L) {
printf("Please input the elements of the list: ");
int e;
while (scanf("%d", &e) == 1) {
ListInsert(L, L->length + 1, e);
}
}
void PrintList(const SqList *L) {
printf("The elements of the list are: ");
for (int i = 0; i < L->length; ++i) {
printf("%d ", L->elem[i]);
}
printf("\n");
}
int main() {
SqList L;
if (!InitList(&L)) {
printf("Failed to initialize the list.\n");
return 0;
}
InputList(&L);
PrintList(&L);
return 0;
}
```
输出元素:
```c
#include <stdio.h>
#include <stdlib.h>
#define LIST_INIT_SIZE 10
#define LIST_INCREMENT 5
typedef struct {
int *elem;
int length;
int listsize;
} SqList;
int InitList(SqList *L) {
L->elem = (int *) malloc(LIST_INIT_SIZE * sizeof(int));
if (!L->elem) {
return 0;
}
L->length = 0;
L->listsize = LIST_INIT_SIZE;
return 1;
}
int ListInsert(SqList *L, int i, int e) {
if (i < 1 || i > L->length + 1) {
return 0;
}
if (L->length >= L->listsize) {
int *newbase = (int *) realloc(L->elem, (L->listsize + LIST_INCREMENT) * sizeof(int));
if (!newbase) {
return 0;
}
L->elem = newbase;
L->listsize += LIST_INCREMENT;
}
int *p = &L->elem[i - 1];
for (int *q = &L->elem[L->length - 1]; q >= p; --q) {
*(q + 1) = *q;
}
*p = e;
++L->length;
return 1;
}
void InputList(SqList *L) {
printf("Please input the elements of the list: ");
int e;
while (scanf("%d", &e) == 1) {
ListInsert(L, L->length + 1, e);
}
}
void PrintList(const SqList *L) {
printf("The elements of the list are: ");
for (int i = 0; i < L->length; ++i) {
printf("%d ", L->elem[i]);
}
printf("\n");
}
void ReverseList(SqList *L) {
for (int i = 0, j = L->length - 1; i < j; ++i, --j) {
int temp = L->elem[i];
L->elem[i] = L->elem[j];
L->elem[j] = temp;
}
}
int main() {
SqList L;
if (!InitList(&L)) {
printf("Failed to initialize the list.\n");
return 0;
}
InputList(&L);
PrintList(&L);
ReverseList(&L);
printf("After reversing, ");
PrintList(&L);
return 0;
}
```
需要注意的是,动态顺序表需要在使用完毕后及时释放内存。可以在程序末尾添加以下语句:
```c
free(L.elem);
```
阅读全文
相关推荐

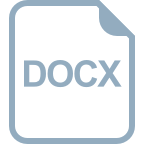
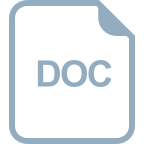



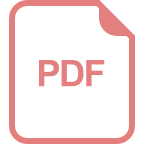
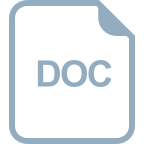
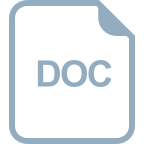
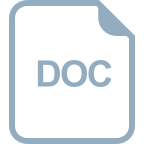



