帮忙写一个c++代码 内容是实现五个字符串要求他们从小到大顺序排序用string方法
时间: 2023-04-03 17:01:57 浏览: 126
好的,以下是实现五个字符串从小到大排序的 C 代码,使用了 string 方法:
```
#include <stdio.h>
#include <string.h>
int main() {
char str[5][20], temp[20];
int i, j;
printf("请输入五个字符串:\n");
for (i = ; i < 5; i++) {
scanf("%s", str[i]);
}
for (i = ; i < 4; i++) {
for (j = i + 1; j < 5; j++) {
if (strcmp(str[i], str[j]) > ) {
strcpy(temp, str[i]);
strcpy(str[i], str[j]);
strcpy(str[j], temp);
}
}
}
printf("排序后的字符串为:\n");
for (i = ; i < 5; i++) {
printf("%s\n", str[i]);
}
return ;
}
```
相关问题
对字符串年月日进行从小到大排序的c++代码
以下是对字符串年月日进行从小到大排序的C代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_DATE_LEN 11
int compare_dates(const void *a, const void *b) {
char *date1 = *(char **)a;
char *date2 = *(char **)b;
int year1, month1, day1;
int year2, month2, day2;
sscanf(date1, "%d-%d-%d", &year1, &month1, &day1);
sscanf(date2, "%d-%d-%d", &year2, &month2, &day2);
if (year1 != year2) {
return year1 - year2;
} else if (month1 != month2) {
return month1 - month2;
} else {
return day1 - day2;
}
}
int main() {
char *dates[] = {"2022-01-01", "2021-12-31", "2022-01-02", "2021-12-30"};
int num_dates = sizeof(dates) / sizeof(char *);
qsort(dates, num_dates, sizeof(char *), compare_dates);
for (int i = 0; i < num_dates; i++) {
printf("%s\n", dates[i]);
}
return 0;
}
```
这段代码使用了 `qsort` 函数来对字符串数组进行排序,排序的依据是年月日的大小关系。函数 `compare_dates` 用来比较两个日期字符串的大小,它首先将日期字符串解析成年月日三个整数,然后按照年、月、日的顺序比较它们的大小。最后,主函数中调用 `qsort` 函数对日期字符串数组进行排序,并输出排序后的结果。
本题要求编写程序,将给定字符串去掉重复的字符后,按照字符ASCII码顺序从小到大排序后输出。 输入格式: 输入是一个以回车结束的非空字符串(少于80个字符)。 输出格式: 输出去重排序后的结果字符串。(用c++实现)
好的,以下是使用 C++ 实现的代码:
```c++
#include<iostream>
#include<set>
#include<algorithm>
using namespace std;
int main() {
string s;
getline(cin, s); // 获取输入字符串
s.erase(remove_if(s.begin(), s.end(), [](const char &c) { // 去重
return count(s.begin(), s.end(), c) > 1;
}), s.end());
sort(s.begin(), s.end()); // 排序
cout << s << endl; // 输出结果
return 0;
}
```
这个程序首先使用 `getline()` 函数获取用户输入的字符串,并存储在 `s` 变量中。然后使用 `erase()` 函数和 `remove_if()` 算法去重,其中 `remove_if()` 算法接受一个 lambda 表达式,通过统计字符在字符串中出现的次数来判断是否重复。接下来使用 `sort()` 函数对字符串进行排序。最后输出去重排序后的结果字符串。
请注意,如果要处理多个输入字符串,可以将上述代码放在一个循环中,重复执行即可。同时需要注意,`getline()` 函数会读取输入行的所有字符,包括回车符,因此需要使用 `erase()` 函数去除回车符。
阅读全文
相关推荐
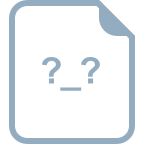













