matlab 区域生长法分割灰度不均匀图像代码
时间: 2023-09-23 12:13:31 浏览: 128
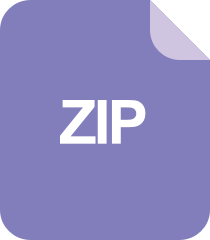
区域生长法图像分割代码
区域生长法是一种基于相似性的图像分割方法,可以用于分割灰度不均匀的图像。下面是一个使用 MATLAB 实现的区域生长法分割灰度不均匀图像的代码示例:
```matlab
% 读入需要分割的图像
img = imread('image.jpg');
% 将图像转换为灰度图像
gray_img = rgb2gray(img);
% 初始化分割结果图像
seg_img = zeros(size(gray_img));
% 设置阈值,用于控制生长的过程
threshold = 30;
% 设置生长的起始点
seed = [100, 100];
% 定义生长的邻域大小
neighborhood_size = 8;
% 定义生长的标记矩阵,用于标记已经被分配到哪个区域
label = zeros(size(gray_img));
% 定义区域生长的条件函数
condition = @(x, y, seed_value) abs(double(gray_img(x, y)) - double(seed_value)) < threshold;
% 定义区域生长的操作函数
operation = @(x, y, seed_value) label(x, y) = seed_value;
% 进行区域生长
[seg_img, label] = region_growing(gray_img, seg_img, seed, neighborhood_size, condition, operation, label);
% 显示分割结果
imshow(seg_img);
```
上述代码中,使用了 `region_growing` 函数进行区域生长。该函数的代码如下:
```matlab
function [seg_img, label] = region_growing(img, seg_img, seed, neighborhood_size, condition, operation, label)
% 获取图像的宽度和高度
img_width = size(img, 2);
img_height = size(img, 1);
% 将种子点加入队列中
queue = [seed(1), seed(2)];
% 标记种子点所在的区域
seed_value = img(seed(1), seed(2));
label(seed(1), seed(2)) = seed_value;
% 遍历队列中的像素
while ~isempty(queue)
% 取出队列中的第一个像素
current_pixel = queue(1,:);
queue(1,:) = [];
% 获取当前像素的坐标
current_x = current_pixel(1);
current_y = current_pixel(2);
% 判断当前像素是否已经被分配到区域中
if seg_img(current_x, current_y) == 0
% 将当前像素分配到区域中
seg_img(current_x, current_y) = 255;
% 判断当前像素的邻域像素是否满足条件,满足条件则加入队列中
for i = current_x-neighborhood_size:current_x+neighborhood_size
for j = current_y-neighborhood_size:current_y+neighborhood_size
% 判断像素是否越界
if i < 1 || i > img_height || j < 1 || j > img_width
continue;
end
% 判断像素是否已经被分配到区域中
if seg_img(i, j) ~= 0
continue;
end
% 判断像素是否满足条件
if condition(i, j, seed_value)
seg_img(i, j) = 255;
queue(end+1,:) = [i, j];
operation(i, j, seed_value);
end
end
end
end
end
```
在 `region_growing` 函数中,首先将种子点加入到队列中,并标记该点所在的区域。然后,遍历队列中的像素,将符合条件的邻域像素加入到队列中,并将这些像素分配到区域中。最后返回分割结果图像和标记矩阵。
需要注意的是,此处的代码仅适用于分割灰度不均匀的图像,对于分割彩色图像需要进行适当的修改。
阅读全文
相关推荐
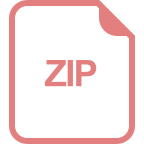
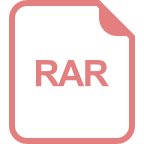
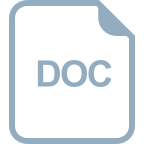
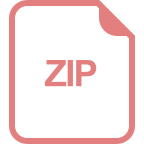
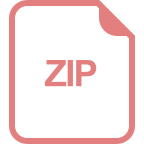
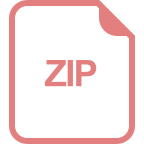
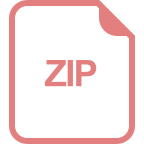
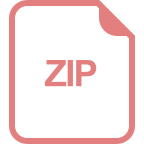
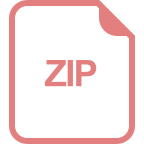
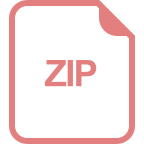
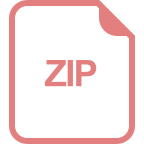
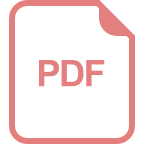
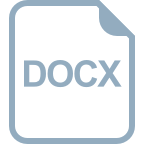
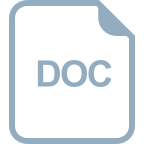
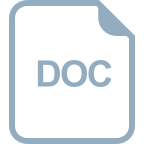
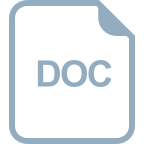
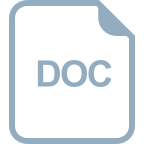