set list map 的使用场景
时间: 2023-09-24 14:11:53 浏览: 95
set、list、map 都是常用的数据结构,它们在不同的场景下有不同的使用方式。
set 是一个不允许重复元素的集合,适合用来进行去重操作,例如统计某个字符串中不同字符的个数,或者求两个数组的交集、并集等。
list 是一个可变长度的有序列表,适合用来存储一组元素,例如用来存储日志信息、任务列表等。
map 是一个键值对的集合,可以通过键快速访问到对应的值,适合用来存储需要按照某个关键字进行索引的数据,例如存储学生的成绩、统计单词出现的频率等。
需要根据具体的需求来选择使用哪种数据结构,或者在不同的数据结构之间进行转换。
相关问题
set list map 区别
set、list和map是不同类型的数据结构,主要区别如下:
1. Set:集合类型,其中不允许有重复元素,常用于去重操作。Set中的元素没有顺序,不能通过下标访问。
2. List:列表类型,其中可以有重复元素,元素按照插入顺序排序,可以通过下标访问。
3. Map:字典类型,存储键值对,键是唯一的,值可以重复。可以通过键来访问值。
总的来说,Set、List、Map在数据结构上的区别主要在于元素的存储方式和访问方式。Set是无序的,List是有序的,Map是通过键值对来访问元素的。在实际应用中,根据不同的需求和场景,选择合适的数据结构可以提高代码的效率和可读性。
set list map区别
set、list、map 都是常见的数据结构,它们的主要区别在于存储方式和使用场景。
set 是一种无序的、不重复的数据结构,它可以用来存储一组互不相同的元素,常用于去重和判重。
list 是一种有序的、可重复的数据结构,它可以用来存储一组有序的元素,常用于队列和栈等数据结构的实现。
map 是一种键值对的数据结构,它可以用来存储一组键值对,常用于快速查找和索引。
总的来说,set 适用于需要去重或判重的场景,list 适用于需要有序存储的场景,map 适用于需要快速查找和索引的场景。
阅读全文
相关推荐
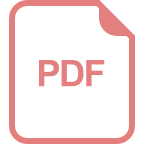
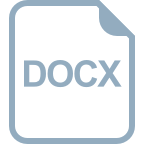
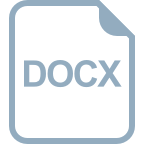
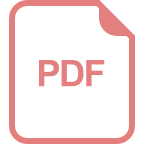
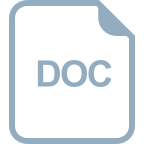
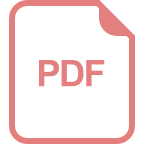
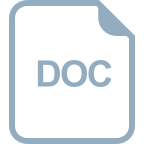
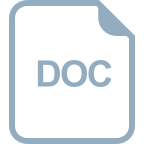
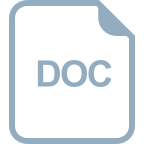
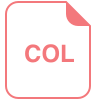



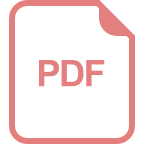
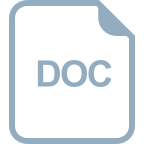
