用java写一个备份文件的代码
时间: 2024-12-22 22:18:25 浏览: 3
在Java中,我们可以创建一个简单的工具类来备份文件。下面是一个基本的示例,它使用`java.io`包里的`FileInputStream`、`FileOutputStream`和`FileUtils`来自Apache Commons IO库来进行文件复制操作。首先,确保已添加了Apache Commons IO依赖。
```java
import org.apache.commons.io.FileUtils;
public class FileBackup {
public static void backupFile(String sourcePath, String destinationPath) throws Exception {
// 检查源文件是否存在
if (!new File(sourcePath).exists()) {
throw new FileNotFoundException("Source file does not exist at " + sourcePath);
}
// 创建目标目录如果不存在
if (!new File(destinationPath).mkdirs() && !new File(destinationPath).isDirectory()) {
throw new IOException("Failed to create directory at " + destinationPath);
}
// 开始备份过程
try (FileInputStream in = new FileInputStream(sourcePath);
FileOutputStream out = new FileOutputStream(destinationPath)) {
FileUtils.copyFile(in, out);
System.out.println("File backed up successfully from " + sourcePath + " to " + destinationPath);
} catch (IOException e) {
System.err.println("Error occurred while backing up file: " + e.getMessage());
}
}
}
// 调用示例
public static void main(String[] args) {
try {
FileBackup.backupFile("path/to/source/file", "path/to/backup/directory");
} catch (Exception e) {
e.printStackTrace();
}
}
```
阅读全文
相关推荐
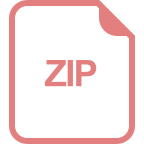
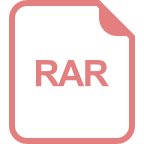
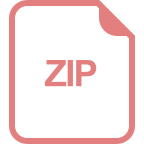
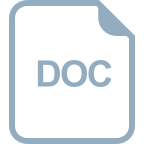
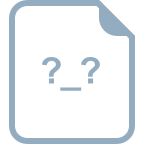
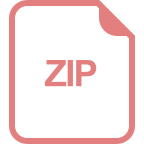
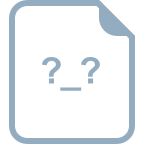
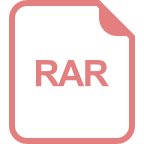
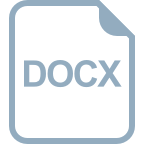
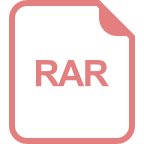
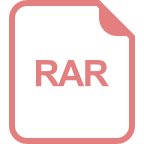
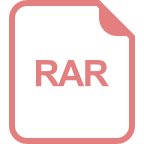
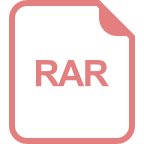
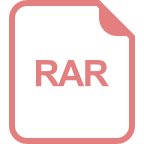
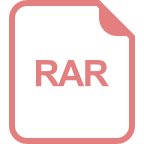
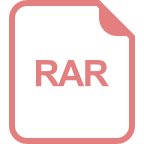
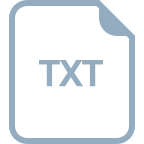
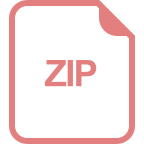
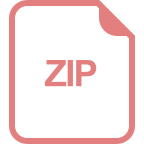