Qt中如何有效的比对字符串
时间: 2024-10-17 12:10:39 浏览: 23
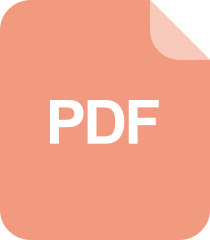
QT中字符串的比较、查找、替换等操作 - 大存的博客1
在Qt中,可以使用多种方法有效地比较字符串。其中最常用的是`QString`类提供的成员函数:
1. **使用** `==` **运算符**: 如果你想检查两个字符串是否完全匹配,可以直接使用`==`运算符,如`if (str1 == str2)`。
```cpp
QString str1 = "Hello";
QString str2 = "Hello";
if (str1 == str2) {
qDebug() << "Strings are equal.";
}
```
2. **使用** `.startsWith()` **和** `.endsWith()**: 这些函数分别用于检查字符串是否以某个子串开始或结束。例如:
```cpp
if (str1.startsWith("Hi")) {
// do something
}
```
3. **使用** `.contains()` **或** `.indexOf()**: 这些函数可以查找子串在原字符串中的位置。比如:
```cpp
int index = str1.indexOf("World");
if (index != -1) {
// substring found
}
```
4. **QRegExp 和正则表达式**: 如果你需要进行更复杂的模式匹配,可以使用`QRegExp`库,它支持正则表达式。例如:
```cpp
QRegExp regex("\\d+");
bool match = regex.exactMatch(str1);
if (match) {
// matches one or more digits
}
```
5. **自定义比较函数**: 通过实现`qstrcmp()`或重载`<`, `>`, `<=`, `>=`等运算符,你可以创建自定义的字符串比较规则。
```cpp
bool compareCaseInsensitive(const QString &str1, const QString &str2) {
return str1.toLower() == str2.toLower();
}
if (compareCaseInsensitive(str1, str2)) {
// strings are equal ignoring case
}
```
阅读全文
相关推荐
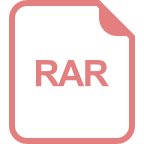
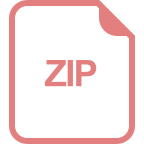
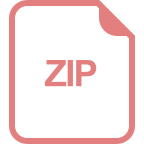
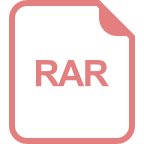
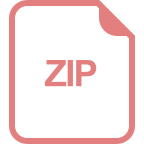
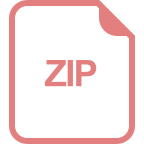
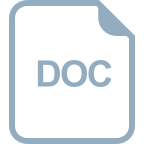
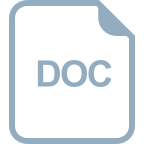



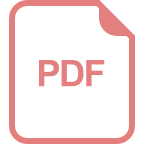
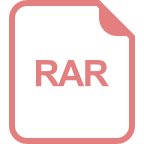
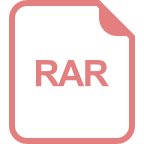
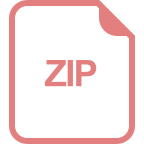
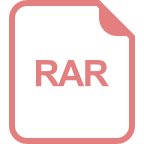
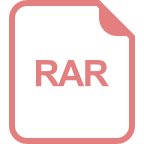