如何在程序中设计报错
时间: 2024-09-19 17:16:01 浏览: 42
在Python中,设计合理的错误处理是编程的重要组成部分,可以帮助提高代码的健壮性和用户体验。以下是在程序中设计报错的一些基本步骤:
1. **使用try/except块**:当你预计可能会发生错误的代码行或操作时,你可以将其放在`try`语句块内。如果这段代码执行失败(即引发异常),则控制权会被转移至对应的`except`块。
```python
try:
# 可能会抛出异常的代码
result = some_function_that_may_fail()
except ExceptionType as e:
# 处理特定类型的异常
print(f"An error occurred: {e}")
```
这里的`ExceptionType`是你希望捕获的具体异常类型,如`ValueError`, `TypeError`, 或自定义的异常。
2. **使用多个except块**:如果你希望处理不同类型的错误,可以在`except`后面跟上具体的异常类型,也可以用`Exception`来捕获所有未指定的异常。
```python
try:
...
except ValueError:
...
except TypeError:
...
except Exception as e:
# 捕获所有其他类型的异常
print("A general error occurred:", str(e))
```
3. **使用finally块**:无论是否发生异常,`finally`块中的代码都会被执行,通常用于清理资源或执行必须完成的操作。
```python
try:
...
except:
...
finally:
# 这里总是会执行,无论之前是否有异常
file.close() # 关闭文件
```
4. **自定义异常**:如果你想创建自己的错误类型,可以继承`Exception`基类并定义一个新的类。这有助于提供更有针对性的错误信息。
```python
class MyCustomError(Exception):
def __init__(self, message):
self.message = message
super().__init__(self.message)
try:
if condition:
raise MyCustomError("This is a custom error.")
except MyCustomError as e:
print(e)
```
5. **记录和报告错误**:考虑使用日志库,如`logging`,记录详细的错误信息,以便于后期分析。
```python
import logging
logger = logging.getLogger(__name__)
logger.error("An unexpected error occurred: %s", exc_info=True)
# 使用文件handler保存到文件
fh = logging.FileHandler('error_log.txt')
fh.setLevel(logging.ERROR)
formatter = logging.Formatter('%(asctime)s - %(levelname)s - %(message)s')
fh.setFormatter(formatter)
logger.addHandler(fh)
```
阅读全文
相关推荐





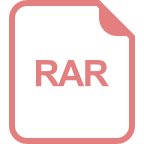












