请你阅读这个文档,按照文中的算法,分别给出使用三次样条拟合积分,高斯勒让德积分,三次样条结合高斯勒让德积分方法的MATLAB代码
时间: 2024-10-15 09:24:45 浏览: 42
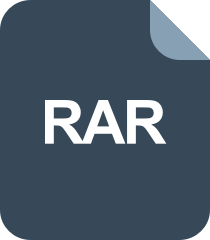
拉格朗日、龙贝格、龙格库塔、三次样条的算法实现

以下是基于文中所述算法,使用MATLAB实现三种积分方法的示例代码框架:
### 三次样条拟合积分方法
```matlab
function result = cubicSplineIntegration(f, J_m, rho, k_rho_min, k_rho_max, n_intervals)
% ... 参数初始化 ...
h = (k_rho_max - k_rho_min) / n_intervals;
X = @(k_rho) f(k_rho) .* J_m(k_rho * rho) .* k_rho.^n;
% 初始化积分结果数组
integral_result = 0;
for j = 1:n_intervals
k_rho_j = k_rho_min + j*h;
% 使用中心差商估计导数
M_j = (X(k_rho_j + h) - X(k_rho_j - h)) / (2*h);
% 计算多项式系数
C_j_3 = (M_j+1 - M_j) / (6*h^2);
C_j_2 = (k_rho_j+1*M_j+1 - k_rho_j*M_j) / (2*h^2);
C_j_1 = (M_j - M_j+1) / h + (k_rho_j+1^2*M_j+1 - k_rho_j^2*M_j) / (2*h);
C_j_0 = X(k_rho_j) - (C_j_3*k_rho_j^3 + C_j_2*k_rho_j^2 + C_j_1*k_rho_j);
% 计算当前区间上的积分
interval_integral = (C_j_3*k_rho_j^4/4 + C_j_2*k_rho_j^3/3 + C_j_1*k_rho_j^2/2 + C_j_0*k_rho_j);
% 累加区间积分结果
integral_result = integral_result + interval_integral;
end
result = integral_result;
end
```
### 高斯-勒让德积分方法
```matlab
function result = gaussLegendreIntegration(f, J_m, rho, k_rho_min, k_rho_max, n_points)
% ... 参数初始化 ...
% 获取高斯-勒让德权重和节点
[weights, nodes] = gausslegendre(n_points);
integral_result = 0;
for l = 1:n_points
t_l = nodes(l);
W_l = weights(l);
% 变量替换
k_rho_transformed = (k_rho_max - k_rho_min)/2 * t_l + (k_rho_max + k_rho_min)/2;
% 计算被积函数值
function_value = f(k_rho_transformed) .* J_m(k_rho_transformed * rho) .* k_rho_transformed^n;
% 更新积分结果
integral_result = integral_result + W_l * function_value;
end
result = integral_result * (k_rho_max - k_rho_min)/2;
end
% Gauss-Legendre quadrature points and weights generation (simplified version)
function [weights, nodes] = gausslegendre(N)
% This is a simplified placeholder function.
% In practice, use precomputed values or numerical methods to find the roots of the Nth Legendre polynomial.
% For demonstration purposes, we'll just return dummy data.
weights = ones(1,N) / N; % Equal weighting as an approximation
nodes = linspace(-1,1,N); % Linearly spaced nodes as an approximation
end
```
### 三次样条结合高斯-勒让德积分方法
```matlab
function result = combinedIntegration(f, J_m, rho, k_rho_min, k_rho_max, n_intervals, n_gauss_points)
% ... Parameters initialization ...
smooth_part_integral = cubicSplineIntegration(@(k_rho) f(k_rho), J_m, rho, k_rho_min, k_rho_max, n_intervals);
oscillatory_part_integral = gaussLegendreIntegration(@(k_rho) f(k_rho) .* J_m(k_rho*rho).^3 .* k_rho^(n+3), 1, rho, k_rho_min, k_rho_max, n_gauss_points);
result = smooth_part_integral + oscillatory_part_integral;
end
```
请注意,这里的代码仅作为概念性示例,实际应用中可能需要更多的细节调整和完善。此外,`gausslegendre` 函数在这里进行了简化处理,实际使用时应依据具体需求选择或实现适当的方法获取精确的高斯-勒让德积分点和权重。
阅读全文
相关推荐
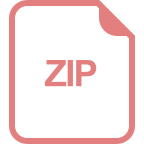
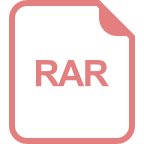
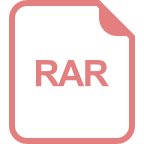
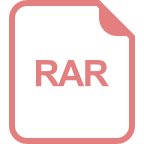
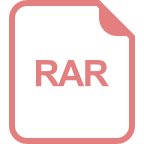
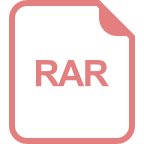
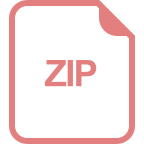
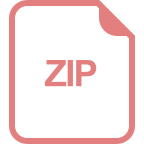
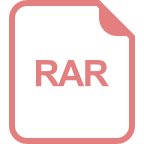
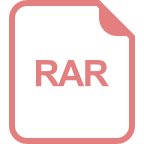
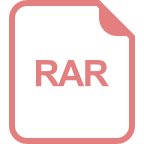
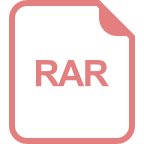
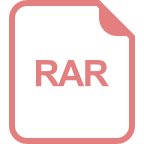
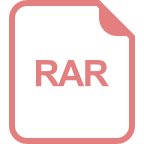
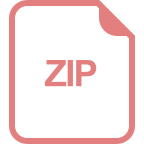
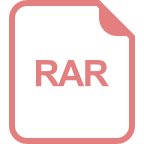
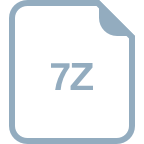